Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial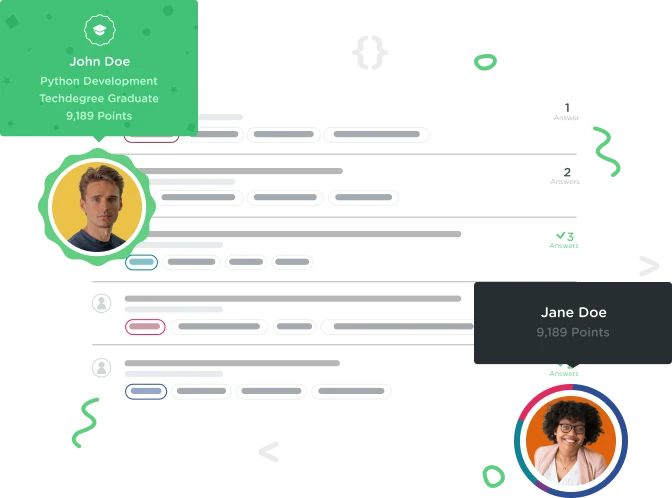
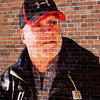
Arthur Wells
9,520 PointsWhen is the function called?
I am certainly able to write the code and get the results, but it isn't making sense as to why it works...
What I don't understand is when is the function being invoked, and how it is actually being called twice; once for guess and once for randomNumber?
The top for lines define vars. Then a function is defined, but not called.
Then we get to the while loop. First we have the condition (guess !== randomNumber). I understand that guess was set to zero, but where is the value for randomNumber coming from...the function hasn't been called so no value has been generated, so I accept that that's ok, at this point because this is just a condition yet to be applied.
The we come to: guess = getRandomNumber(upper);
Now this appears to be just resetting the variable value, not calling the getRandomNumber() function. However, even if I accept that this is actually calling the getRandomNumber() function, then I now have one getRandomNumber() value [and one randomNumber value], and it is the one being assigned to guess. It looks like the function has only been called once and if that is so, and both guess and randomNumber are using it then they should match immediately.
Clearly I'm missing something, likely simple.
Can someone explain this clearly to me?
Thanks!
4 Answers
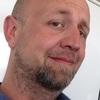
Shawn Denham
Python Development Techdegree Student 17,801 PointsYou are pretty much there. If we break it down step by step it makes sense. Forgive me if I go to basic on you but I am not completely clear on what you are ok on and what you are having trouble with so I am just going to try and cover it all.
This is going to be super long but please be patient and read it step by step while you are looking at the code!
The script:
var upper 10000;
var randomNumber = getRandomNumber(upper);
var guess;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber( upper );
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p> It took the computer " + attempts + " attempts to get it right.</p>");
So when the page loads JavaScript
- Scans the script : This is where all of the variables are initialized and functions are read and stored into memory.
- Execute the script : Start the line by line execution of the script,
- set the value of upper to 10000
- set the value of randomNumber to the value that gets returned from the function getRandomNumber() (lets assume it returned 107 so as of right now guess = 0 and randomNumber = 107)
- nothing to do with guess right now we have already initialized it and no value is being assigned to it right now.
- set the value of attempts to 0
- evaluate the while loop: is it true that the value of guess (0) is not equal to the value of randomNumber(107)? yes that is true..so
- run the code in the while loop lets call the function getRandomNumber and assign that value to guess. (lets assume that the function getRandomNumber returned a value of 286) so now guess = 286 and randomNumber still = 107**
- increment the value of attempts by 1 so now attempts = 1.
whew! ok we have gone though everything once now and here is where we stand
- randomNumber = 107
- guess = 286
- attempts = 1
Since our first run through the while loop evaluated to true we ran through the code in the loop now we need to evaluate our condition again! so....
- evaluate the while loop: is it true that the value of guess (which is now 286, remember?) is not equal to the value of randomNumber (which is still 107)? YES! this is true. 286 does not equal 107. So since this evaluated to TRUE (286 does NOT equal 107) we need to run through the while loop code.
- run the code in the while loop lets call the function getRandomNumber again and assign that value to guess. (lets assume that the function getRandomNumber returned a value of 1467) so now guess = 1467 and randomNumber is still = 107**
- increment the value of attempts by 1 so now attempts = 2.
Now we have gone through the loop twice and here is where we stand...
- randomNumber = 107
- guess = 1467
- attempts = 2
Since our last run through the while loop evaluated to true we ran through the code in the loop now we need to evaluate our condition again! so....
- evaluate the while loop: is it true that the value of guess (which is now 1467) is not equal to the value of randomNumber (which is still 107)? YES! this is true. So we need to run through the while loop code again.
- run the code in the while loop lets call the function getRandomNumber again and assign that value to guess. (lets assume that the function getRandomNumber returned a value of 107) so now guess = 107 and randomNumber is still = 107**
- increment the value of attempts by 1 so now attempts = 3.
Now we have gone through the loop three times and here is where we stand...
- randomNumber = 107
- guess = 107
- attempts = 3
Since our last run through the while loop evaluated to true we ran through the code in the loop now we need to evaluate our condition again! so....
- evaluate the while loop: is it true that the value of guess (which is now 107) is not equal to the value of randomNumber (which is still 107)? NO! this is false because 107 DOES equal 107!
Since the evaluation came back as false we break out of the loop (remember, in any loop you stay in the loop as long as the evaluation is true This is the same for WHILE, DO WHILE and FOR loops. As long as the evaluation returns true we keep running the code in the loop!) So now we run the next line of code after the loop..
- write the results to the screen tell the user what the random number was that the computer was trying to "match"
- write to the screen tell the user the number of attempts is took the computer to match or "guess" the number.
I know that was hella long but I hope it helped. If you have a question about a specific step please let me know!
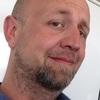
Shawn Denham
Python Development Techdegree Student 17,801 PointsOh and the most important part....The funny thing about this exercise is that once you figure out that the computer can NEVER NEVER EVER guess it right on the first try a lot of things click into place!
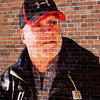
Arthur Wells
9,520 PointsThanks Shawn. Great answer. So if I'm getting it right, it has to run any functions that it requires in order to set variables either when it scans the script or begins execution. I think this is making more sense now. Staying Tuned! Archie
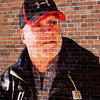
Arthur Wells
9,520 PointsHey, just got part two! Thanks for all the effort. I am looking forward to working through it...even part one was a big help. Archie
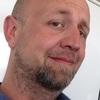
Shawn Denham
Python Development Techdegree Student 17,801 PointsGlad to hear it! I am not sure but if you are having problems getting your head wrapped around how that function works let me know and I'll try to help explain that too!