Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial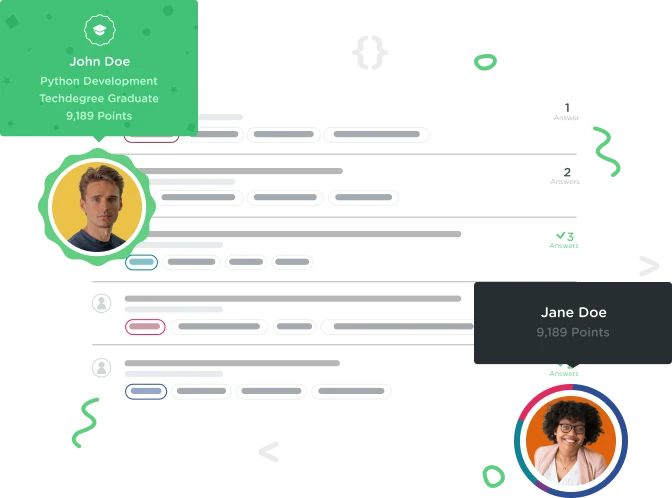

Erik L
Full Stack JavaScript Techdegree Graduate 19,470 Pointswhen my code runs, I see undefined, can someone help?
function print (message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML += message;
}
var HTML = '';
var students = [
{
name: 'Erik',
track: 'Full Stack Javascript',
achievements: 100,
points: 3000
},
{
name: 'Jackie',
track: 'Python',
achievements: '50',
points: '300'
},
{
name: 'Josh',
track: 'CSS',
achievements: '400',
points: '2000'
},
{
name: 'Alicia',
track: 'PHP',
achievements: '5000',
points: '3000'
},
{
name: 'Betty',
track: 'Java',
achievements: '5000',
points: '4000'
}
];
for (var i = 0; i < students.length; i++) {
HTML += '<br>'
HTML += '<b>Student: ' + students[i].Name + '</b><br>';
HTML += 'Track: ' + students[i].Track + '<br>';
HTML += 'Achievements: ' + students[i].Achievements + '<br>';
HTML += 'Points: ' + students[i].Points + '<br>';
}
print(HTML);
4 Answers

KRIS NIKOLAISEN
54,971 PointsFirst off you have an inconsistency with no quotes around the values for achievements and points here:
name: 'Erik',
track: 'Full Stack Javascript',
achievements: 100,
points: 3000
but the reason you see undefined is JavaScript is case sensitive. name, track, achievements and points have all been capitalized here:
HTML += '<br>'
HTML += '<b>Student: ' + students[i].Name + '</b><br>';
HTML += 'Track: ' + students[i].Track + '<br>';
HTML += 'Achievements: ' + students[i].Achievements + '<br>';
HTML += 'Points: ' + students[i].Points + '<br>';
Switch those to lowercase and you should be good.

KRIS NIKOLAISEN
54,971 PointsKristaps, you need a return statement. From MDN:
Every function in JavaScript is a Function object. ... A function without a return statement will return a default value. In the case of a constructor called with the new keyword, the default value is the value of its this parameter. For all other functions, the default return value is undefined

Kristaps Vecvagars
6,193 PointsI see. However, in this case, when the function as tasked to simply push object into an array, what would the return be? Is it considered bad practice not to return anything?

KRIS NIKOLAISEN
54,971 PointsMaybe I misspoke. You don't NEED a return statement. Without one the returned value is undefined.
Functions without return statements are fine. You would just call it without assigning it to anything. If you did assign the result, it would always return the default undefined. Here are stack overflow answers
Where you will find:
If you're returning undefined, specifically, you can just write
return;

Kristaps Vecvagars
6,193 PointsThank you very much for the explanation!

Kristaps Vecvagars
6,193 PointsI am also puzzled about the return of "undefined". I wrote a function that creates objects and pushes them into a predefined, empty array. The function works just fine, however, each time I run it, "undefined" is also returned to the console.
Any hints as to why that is? What am I missing?
function insertStudent(name, track, achievements, points) {
let test_object = {};
test_object.name = name;
test_object.track = track;
test_object.achievements = achievements;
test_object.points = points;
test_array.push(test_object);
};
Erik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsErik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsI was actually wondering if the numbers were supposed to have quotes or not, I followed the video exactly, that is why some numbers haves quotes and some don't