Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial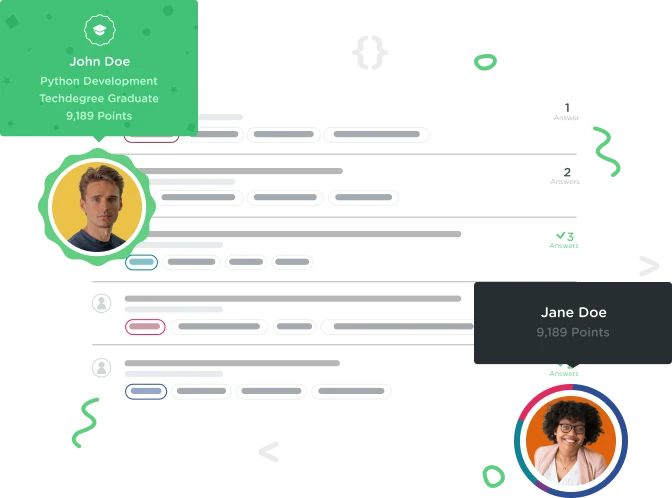
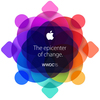
Aryan Kashyap
Courses Plus Student 5,943 PointsWhen setting an Adapter it Gives a Null Pointer Exception
The Line
The code is
The Line
JAVA
The null Pointer Exception is given Around
mealRating.setAdapter(spinnerAdapter)
The Place Where Its Assigned is,,
mealRating = ((Spinner) v.findViewById(R.id.rating_spinner));
`package com.aryan.mealspotter; public class NewMealFragement extends Fragment {
private ImageButton photoButton;
private Button saveButton;
private Button cancelButton;
private TextView mealName;
private Spinner mealRating;
private ParseImageView mealPreview;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup parent,
Bundle SavedInstanceState) {
View v = inflater.inflate(R.layout.activity_new_meal, parent, false);
mealName = ((EditText) v.findViewById(R.id.meal_name));
// The mealRating spinner lets people assign favorites of meals they've
// eaten.
// Meals with 4 or 5 ratings will appear in the Favorites view.
mealRating = ((Spinner) v.findViewById(R.id.rating_spinner));
System.out.println("mealRating object is " + mealRating);
ArrayAdapter<CharSequence> spinnerAdapter = ArrayAdapter
.createFromResource(getActivity(), R.array.ratings_array,
android.R.layout.simple_spinner_dropdown_item);
mealRating.setAdapter(spinnerAdapter);
photoButton = ((ImageButton) v.findViewById(R.id.photo_button));
photoButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
InputMethodManager imm = (InputMethodManager)getActivity()
.getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(mealName.getWindowToken(), 0);
startCamera();
}
});
saveButton = ((Button) v.findViewById(R.id.save_button));
saveButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Meal meal = ((NewMealActivity) getActivity()).getCurrentMeal();
// When the user clicks "Save," upload the meal to Parse
// Add data to the meal object:
meal.setTitle(mealName.getText().toString());
// Associate the meal with the current user
meal.setAuthor(ParseUser.getCurrentUser());
// Add the rating
meal.setRating(mealRating.getSelectedItem().toString());
// If the user added a photo, that data will be
// added in the CameraFragment
// Save the meal and return
meal.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e == null) {
getActivity().setResult(Activity.RESULT_OK);
getActivity().finish();
} else {
Toast.makeText(
getActivity().getApplicationContext(),
"Error saving: " + e.getMessage(),
Toast.LENGTH_SHORT).show();
}
}
});
}
});
cancelButton = ((Button) v.findViewById(R.id.cancel_button));
cancelButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
getActivity().setResult(Activity.RESULT_CANCELED);
getActivity().finish();
}
});
// Until the user has taken a photo, hide the preview
mealPreview = (ParseImageView) v.findViewById(R.id.meal_preview_image);
mealPreview.setVisibility(View.INVISIBLE);
return v;
}
/*
* All data entry about a Meal object is managed from the NewMealActivity.
* When the user wants to add a photo, we'll start up a custom
* CameraFragment that will let them take the photo and save it to the Meal
* object owned by the NewMealActivity. Create a new CameraFragment, swap
* the contents of the fragmentContainer (see activity_new_meal.xml), then
* add the NewMealFragment to the back stack so we can return to it when the
* camera is finished.
*/
public void startCamera() {
Fragment cameraFragment = new CameraFragement();
FragmentTransaction transaction = getActivity().getFragmentManager()
.beginTransaction();
transaction.replace(R.id.fragmentContainer, cameraFragment);
transaction.addToBackStack("NewMealFragment");
transaction.commit();
}
/*
* On resume, check and see if a meal photo has been set from the
* CameraFragment. If it has, load the image in this fragment and make the
* preview image visible.
*/
@Override
public void onResume() {
super.onResume();
ParseFile photoFile = ((NewMealActivity) getActivity())
.getCurrentMeal().getPhotoFile();
if (photoFile != null) {
mealPreview.setParseFile(photoFile);
mealPreview.loadInBackground(new GetDataCallback() {
@Override
public void done(byte[] data, ParseException e) {
mealPreview.setVisibility(View.VISIBLE);
}
});
}
}
}
` Any Help Will Be really really appreciated Thanks Aryan
4 Answers
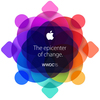
Aryan Kashyap
Courses Plus Student 5,943 PointsBen Jakuben Craig Dennis Please Help As you are The People Who helped me When i was a starter At Android AnyWays Thanks For Helping
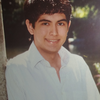
Jon Kussmann
Courses Plus Student 7,254 PointsI would double check your layout and resource xml files to make sure the IDs your supply correspond to your code. You can post them here and I'll help you double check.
Another thing is you might have to set a DropDownViewResouce for your adapter... see the example here: Spinner
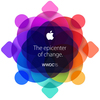
Aryan Kashyap
Courses Plus Student 5,943 PointsHi Jon Kussmann
The Xml Code is
XML
`<?xml version="1.0" encoding="UTF-8"?>
<LinearLayout android:orientation="vertical" android:layout_height="match_parent" android:layout_width="match_parent" xmlns:android="http://schemas.android.com/apk/res/android">
<TextView android:layout_height="wrap_content" android:layout_width="match_parent" android:textSize="20sp" android:text="@string/meal_label" android:layout_marginLeft="5dp"/>
<EditText android:layout_height="wrap_content" android:layout_width="match_parent" android:inputType="textMultiLine" android:id="@+id/meal_name"/>
<Spinner android:layout_height="wrap_content" android:layout_width="wrap_content" android:id="@+id/rating_spinner"/>
<ImageButton android:layout_height="wrap_content" android:layout_width="match_parent" android:id="@+id/photo_button" android:src="@drawable/ic_action_photo"/>
<Button android:layout_height="wrap_content" android:layout_width="match_parent" android:text="@string/save_button_text" android:id="@+id/save_button"/>
<Button android:layout_height="wrap_content" android:layout_width="match_parent" android:text="@string/cancel_button_text" android:id="@+id/cancel_button"/>
<com.parse.ParseImageView android:layout_height="200dp" android:layout_width="wrap_content" android:id="@+id/meal_preview_image"/>
</LinearLayout>`
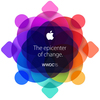
Aryan Kashyap
Courses Plus Student 5,943 PointsWhen I Debugged The App The error was on the line Below mealRating = ((Spinner) v.findViewById(R.id.rating_spinner));
in The class
@Override public View onCreateView(LayoutInflater inflater, ViewGroup parent, Bundle SavedInstanceState) { View v = inflater.inflate(R.layout.activity_new_meal, parent, false);
mealName = ((EditText) v.findViewById(R.id.meal_name));
// The mealRating spinner lets people assign favorites of meals they've
// eaten.
// Meals with 4 or 5 ratings will appear in the Favorites view.
mealRating = ((Spinner) v.findViewById(R.id.rating_spinner));
System.out.println("mealRating object is " + mealRating);
ArrayAdapter<CharSequence> spinnerAdapter = ArrayAdapter
.createFromResource(getActivity(), R.array.ratings_array,
android.R.layout.simple_spinner_dropdown_item);
spinnerAdapter.setDropDownViewResource(android.R.layout.activity_list_item);
mealRating.setAdapter(spinnerAdapter);
photoButton = ((ImageButton) v.findViewById(R.id.photo_button));
photoButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
InputMethodManager imm = (InputMethodManager)getActivity()
.getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(mealName.getWindowToken(), 0);
startCamera();
}
});