Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial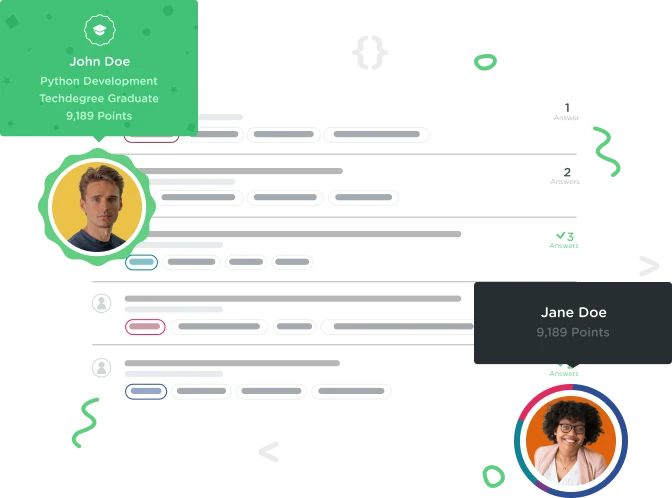

simplyb
27,883 PointsWhen should I use optional channing and when should I use the 'if let' construct?
In the video, the 'if let' construct is used, as well as the optional channing. I tried reading on the Apple docs, but couldn't distinguish when to use which, since they appear to achieve the same outcome. Any thoughts?
Thanks A
2 Answers

Martin Wildfeuer
Courses Plus Student 11,071 PointsOptional chaining is basically the same as optional binding. Optional binding allows you to unwrap a single value, whereas optional chaining allows you to unwrap a property "within" an object. Let's say we have a class called Car
. This car might have extras, but not if it is the "cheap model". Moreover, one of the extras might be airConditioning, but that is not guaranteed. This is how that scenario would look like:
class Car {
var extras: Extras? // Optional, so possibly nil
...
}
class Extras {
var airConditioning: AirConditioning? // Also optional, possibly nil
...
}
class AirConditioning {
var manufacturer: String
...
}
// Let's create a beetle. Extras and Airconditioning might be created afterwards
let beetle = Car()
Let's say we want to get the manufacturer of the airConditioning extra of the Beetle. As both extras and airConditioning are optional, we have to make sure to check it is not nil and unwrap everything one after another:
if let extras = beetle.extras {
if let airConditioning = extras.airConditioning {
let manufacturer = airConditioning.manufacturer
// Now we can use manufacturer
}
}
But we could also access manufacturer "directly", using optional chaining:
if let manufacturer = beetle.extras?.airConditioning?.manufacturer {
// Now we can use manufacturer
}
This code only makes it to the body of the if-statement if extras is not nil and finally airConditioning is not nil.

Ramesh VJ
2,201 PointsThanks for the details explanation.
simplyb
27,883 Pointssimplyb
27,883 PointsWow, such a detailed reply! Thank you so much!