Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial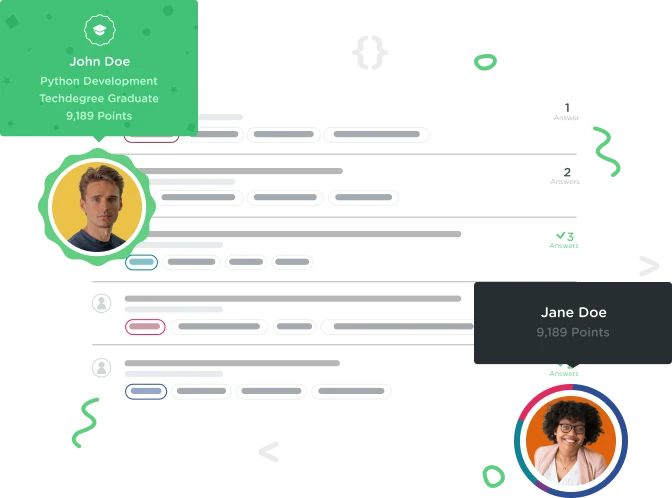
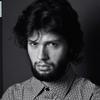
B.B. Groeneveld
2,826 PointsWhen to choose which Logical Operator in this challenge? An AND operator works as well.
Hi everyone,
It seems that my program is running perfectly fine, though I use an AND logical operator in stead of the OR operator, used by Dave in the video. How come it still works? I thought that when using a AND operator, the arguments have to be both isNaN?
Here my written syntax:
// declare program variables
var num1;
var num2;
var message;
// announce the program
alert("Let's do some math!");
// collect numeric input num1
num1 = prompt("Please type a number");
num1 = parseFloat(num1);
//collect numeric input num2
num2 = prompt("Please type another number");
num2 = parseFloat(num2);
//handling error isNaN for input
if ( isNaN( num1 && num2 )) {
alert("At least one of the values you typed is not a number. The page will reload.");
window.location.reload();
}
// handling error 0
if ( num2 === 0 ) {
alert("Division by 0 is not allowed in Math. Please give a correct number.");
window.location.reload();
}
// build an HTML message
message = "<h1>Math with the numbers " + num1 + " and " + num2 + "</h1>";
message += num1 + " + " + num2 + " = " + (num1 + num2);
message += "<br>";
message += num1 + " * " + num2 + " = " + (num1 * num2);
message += "<br>";
message += num1 + " / " + num2 + " = " + (num1 / num2);
message += "<br>";
message += num1 + " - " + num2 + " = " + (num1 - num2);
// write message to web page
document.write(message);
2 Answers

Jacob Mishkin
23,118 PointsYou want both inputs to be a number, and if not throw an error to the user, which you did.
I haven't seen the video, but from the code you are using the OR (||) operator here would not work. The OR operator will check the first expression and if true will execute the code, and will not look at the second expression, unless the first expression is false, then it will look at the second expression.
With the AND (&&) BOTH expressions need to be true in order to execute the code, which you have.
You're good.
Here are some examples using operators:
using &&
a1 = true && true // t && t returns true
a2 = true && false // t && f returns false
a3 = false && true // f && t returns false
using ||
o1 = true || true // t || t returns true
o2 = false || true // f || t returns true
o3 = true || false // t || f returns true

Antti Lylander
9,686 Pointsif ( isNaN( num1 && num2 )) {
alert("At least one of the values you typed is not a number. The page will reload.");
window.location.reload();
alert should be shown when either num1 OR num2 is not a number. Now it will be only shown if both are not numbers. Now, if you only give numbers, everything will seem just fine.

Konrad Dziekonski
7,798 PointsHey
I get a bit cofused in a video there was that this kind of shortcuts cannot be used and it should be
if (isNaN(num1) || isNaN(num2))
so apart from || and && is this:
'if ( isNaN( num1 && num2 ))' and 'if ( isNaN( num1 || num2 ))'
a valid and working piece of code? does the browser execute it this same way as
if (isNaN(num1) || isNaN(num2)) and if (isNaN(num1) && isNaN(num2))
but its just a bad practice?
thanks
Antti Lylander
9,686 PointsAntti Lylander
9,686 PointsHave you tried giving a string (not a number) as an input? Otherwise it does not matter what you put there..