Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial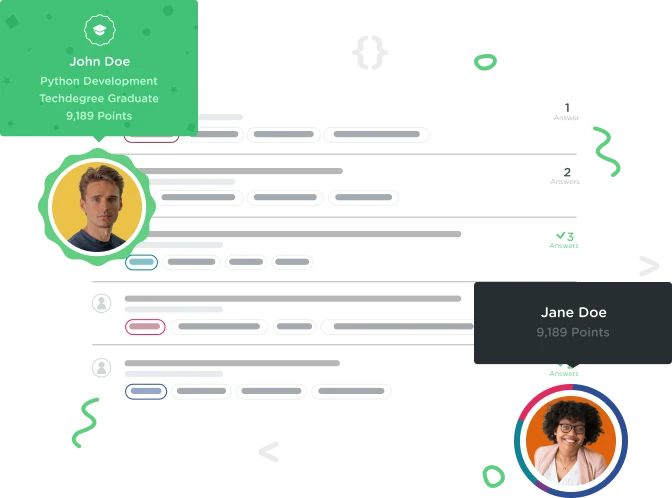

Mikaela Land
Python Web Development Techdegree Student 2,324 PointsWhen to use empty: Strings, lists, dictionaries
So I've been wondering for a while when do you use empty:
strings = " " lists = [ ] dictionaries = { }
and why? I'm just not sure when to use them when I'm programming. Any tips?
2 Answers

Lukas Dahlberg
53,736 PointsStrings are probably the easiest: You'll use an empty one to declare to other programmers that you're expecting the value to hold a string. (As opposed to an integer or a class instance and so on).
For the other two, it really has to do the most typical use case. If you want to maintain order, then you should choose a list as it will reflect the order in which you added (or removed) elements. Whereas you'd want to use a dictionary when you know exactly what you want to call and you don't care where/how it's stored. This means that whereas you'd have to potentially iterate through an entire list to find the element you want, you do not have to do so with a dictionary, leading to faster access of said element. (Python dictionaries are created with a data structure called hash tables - a topic that's very much worth understanding as you grow as programmer, especially if you have any interest in algorithms.)
So in short, use an empty list when you need to track add/remove and a dictionary when you want fast retrieval w/o reference to 'history'.
(As for when to use an empty list versus an existing one, it comes down to what you need to track.)
There are other fine points to discuss in this regard as well, such as space usage, but that will confuse the issue a little bit, so it's best to use the rule of thumb above as you get more comfortable with data structures. As you grow as a developer, you'll start to weigh the pros and cons in total as to which is the right tool for the job.
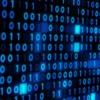
Alexander Davison
65,469 PointsEmpty iterables (strings, dictionaries, lists... etc) are often used, in fact. One common reason is that the iterable is initially empty, because you are going to fill it up with values. For example:
my_list = []
for x in range(100):
my_list.append(x)
print(my_list) # This will print out [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10...] until 100.

Mikaela Land
Python Web Development Techdegree Student 2,324 PointsSo you use empty iterables just to store new things. What about when you already have a used iterable why would you sometimes use and empty interable instead of adding to the old one? I've seen this done a few times, just a little slow to figure it out lately.
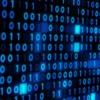
Alexander Davison
65,469 PointsCan you show me an example perhaps? Thanks!

Mikaela Land
Python Web Development Techdegree Student 2,324 PointsActually I can't find one, I've been looking but.... I thought about it perhaps if you need a new separate dictionary or something with different data. I must be losing me mind. Sorry.
I'm in python collections for beginners right now and really struggling with the challenges. I just get confused sometimes about when people add empty interables in their functions. Starting to get it though.