Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial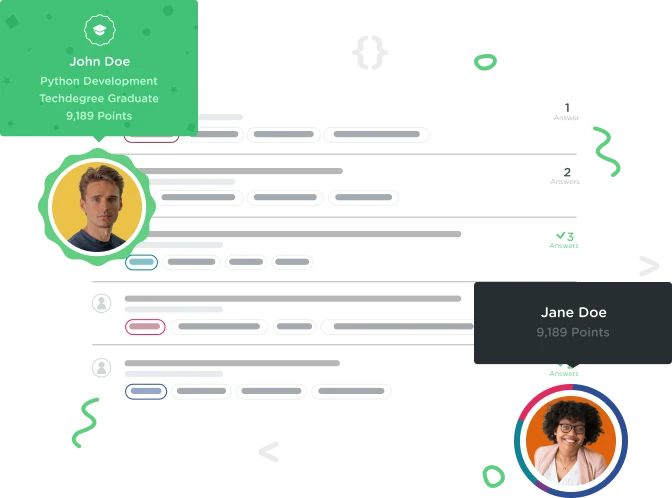

Ivan Yudhi
2,031 PointsWhen to use IllegalArgumentException?
For this part of code exercise:
private char validateGuess(char letter) {
//throw an exception error if not a letter
if (!Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
//convert the letter to lowercase
letter = Character.toLowerCase(letter);
//check if the letter has already been guessed
if(mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed" );
}
return letter;
}
Why do we need to throw IllegalArgumentException? Can't we just do println("message") to display the message, or does it serve other purpose?
1 Answer

Dan Johnson
40,533 PointsThrowing an exception allows you to break out of the current block of code and process the error in a catch
block. In the case of someone passing in something that wasn't a letter, it wouldn't make sense to further process it in the method.
try {
validateGuess(5);
}
catch(IllegalArgumentException e) {
//Would jump from the throw to here.
System.out.println(e.getMessage());
//Do more error handling if needed.
}
//Continue on from here
Dan Johnson
40,533 PointsDan Johnson
40,533 PointsAdded code formatting.