Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial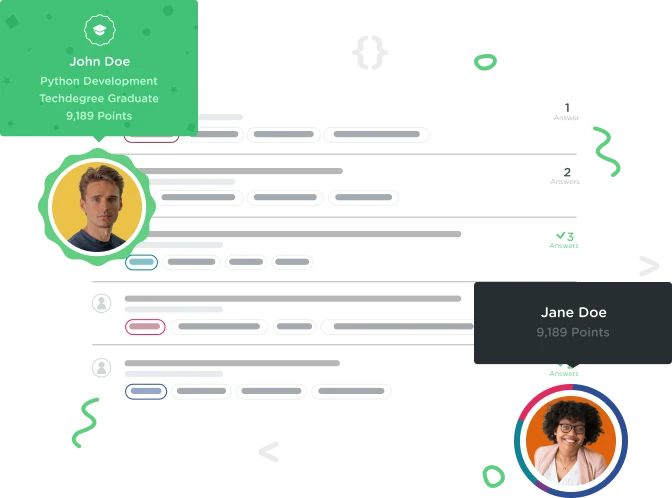
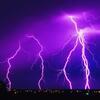
Janelle Mackenzie
7,510 PointsWhen to use quotes in Javascript...?
I understand that single/double quotes are used for strings, and backticks are used for template literals. What I don't understand is the consistency rules of when quotes are required in javascript. I always thought that quotes were only needed when defining a string, so if you were accessing a function or variable, quotes would not be used because functions and variables are not strings. But below is an example contradicting that...
As you can see in the <script> tag, the function addItem has a line with const item = 'userInput';. userInput is not a string, it is a variable I defined at the beginning of my javascript. But when I remove the quotes the code does not work. Can somebody explain why I need the quotes for this variable in order for my code to run? Thanks :)
<!DOCTYPE html>
<html>
<head>
<title>Javascript List</title>
<link rel='stylesheet' href='js-list.css'/>
</head>
<body>
<main>
<h1 class='title'>Grocery List</h1>
<p>Your personal grocery list, add/remove items at your liking!</p>
<input id='user-input' placeholder='ex; bananas'></input>
<button id='user-submit'>ADD TO LIST</button>
<h2 class='heading'>Your Items:</h2>
<ul id='list-items'>
<!-- LIST INSERTED VIA JAVASCRIPT -->
</ul>
<script>
const list = document.getElementById('list-items');
const userInput = document.getElementById('user-input').value;
const addBtn = document.getElementById('user-submit');
const added = addBtn.addEventListener('click', addItem);
function addItem () {
const li = document.createElement('li');
const item = 'userInput';
const x = document.createTextNode(item);
li.appendChild(x);
if (item === '') {
alert('Enter an item to add to the list.');
} else {
list.appendChild(li);
}
}
</script>
</main>
</body>
</html>
1 Answer

Matik Zed
11,533 PointsHello Janelle. I was just playing around with the code. If you pass in item you are literally passing in the string 'userInput' so no matter what I type in the user input bar the string 'userInput' will be appended. I am assuming that what you want is whatever is typed in the user input to be appended to the page. What is happening is that you are defining the value of the user input when the page is loaded. When the page is loaded the user has not put anything into the user input bar, therefore the value of userInput is an empty string. There is no connection between these two lines of code:
const userInput = document.getElementById('user-input').value;
const item = 'userInput';
This looks confusing, because it looks as if userInput the variable and the string 'userInput' may somehow be connected, but they are not. Like I mentioned earlier userInput is an empty string by the time the function addItem runs because the variable is initialized as soon as the page loads. item is literally a string with 'userInput' as it's value. The current code will add this string to the page no matter what I type in the user input. This can be verified by running the code and playing with it. You mentioned that the code would not work if you changed this line of code:
const item = 'userInput';
into this:
const item = userInput;
The second line of code actually does work, except it isn't what you expected it to be. The second line of code is appending the empty string that we were talking about. item is assigned the value of userInput and userInput has an empty string as its value.
so how do we fix this?
If we check the value of the user input in the function instead of the beginning when the page loads, then we can read the actual input the user typed by the time they click the 'ADD TO LIST' button.
<!DOCTYPE html>
<html>
<head>
<title>Javascript List</title>
<link rel='stylesheet' href='js-list.css'/>
</head>
<body>
<main>
<h1 class='title'>Grocery List</h1>
<p>Your personal grocery list, add/remove items at your liking!</p>
<input id='user-input' placeholder='ex; bananas'></input>
<button id='user-submit'>ADD TO LIST</button>
<h2 class='heading'>Your Items:</h2>
<ul id='list-items'>
<!-- LIST INSERTED VIA JAVASCRIPT -->
</ul>
<script>
const list = document.getElementById('list-items');
const addBtn = document.getElementById('user-submit');
const added = addBtn.addEventListener('click', addItem);
function addItem () {
const li = document.createElement('li');
const userInput = document.getElementById('user-input').value;
const x = document.createTextNode(userInput);
li.appendChild(x);
if (userInput === '') {
alert('Enter an item to add to the list.');
} else {
list.appendChild(li);
}
}
</script>
</main>
</body>
</html>
Janelle Mackenzie
7,510 PointsJanelle Mackenzie
7,510 PointsThanks so much! Makes total sense, great explanation :)