Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial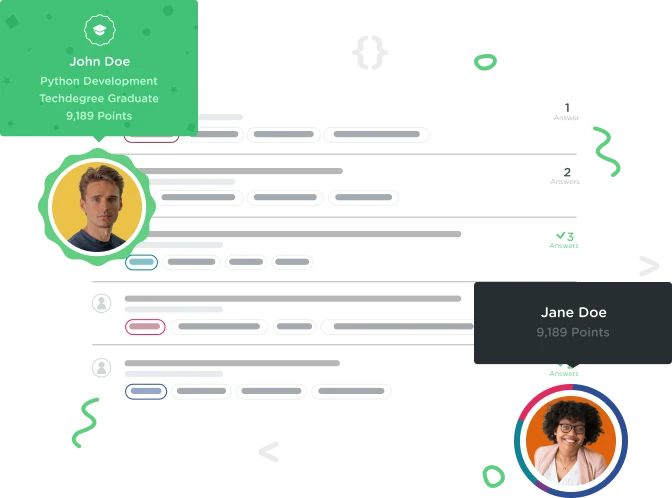
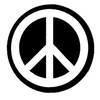
john larson
16,594 Pointswhen to use "return"
the method "equals" uses return but the methods "add" and "this" do not. Why would that be? Or, when is return used or not used in a function.
var calculator = {
sum: 0,
add: function(value) {
this.sum += value;
},
clear: function() {
this.sum = 0;
},
equals: function() {
return this.sum;
}
}
calculator.add(5)
calculator.add(5)
calculator.add(5)
calculator.equals()
3 Answers
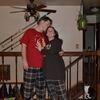
Julie Myers
7,627 PointsThere are two general ways in which to use functions:
(1) The function only does work behind the scenes. Thus, there is no reason for the function to return any data to the caller.
(2) The function needs to not only do some work, but also return data to the caller.
For example:
//Returning a value to the caller:
function returnData(num1, num2) {
return num1 + num2;
}
var saveReturnedData = returnData(4, 5); //returns 9
console.log(saveReturnedData); // writes 9 to the console
//I just need the function to update a variable, NOT return anything:
var name = "Jason";
console.log(name); //"Jason"
function updateName(newName) {
name = newName;
}
updateName("Allen"); // changes the value of the name variable from Jason to Allen.
console.log(name); //"Allen"
There are many scenarios that would dictate when you would want a function to just update data without returning any data, and when you would need a function to return data. When you look at other people's coding take note of when their function returns data and when it doesn't. As you write your own coding ask yourself does your function need to return data, and this will help you get good at it.
The return statement also exit's a function immediately after it has returned it's data. So, if you have any coding, that is within the function, that is after the return statement it will never get run.
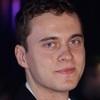
Adrian Patrascu
15,279 PointsHi John,
In this case the "add" method you have used needs just to add 2 numbers, there is no need to return them as you have another method that is equivalent to the equal sign, and thus use that method as a return. Return should be used when you want to exit the function and return a value from it. For a reference please see: http://www.w3schools.com/jsref/jsref_return.asp
Thank you, Adrian
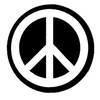
john larson
16,594 PointsThank you Adrian. So then "this.sum = 0;", the equals sign acts the same way as the return keyword?
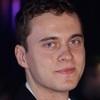
Adrian Patrascu
15,279 PointsWell kind off in this particular case, this is more of "reset" meaning it makes the sum 0. It is more like making the sum equal to 0. You do not need all functions to be used with a return statement, some of them are intermediate steps until you can get yo your result.
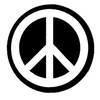
john larson
16,594 PointsThank you Julie and Adrian, that should clear it up for me.
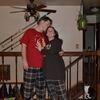
Julie Myers
7,627 PointsJust to add to what Adrian said, if a function does not have a return statement then it always returns undefined. Just the way javaScript works.