Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial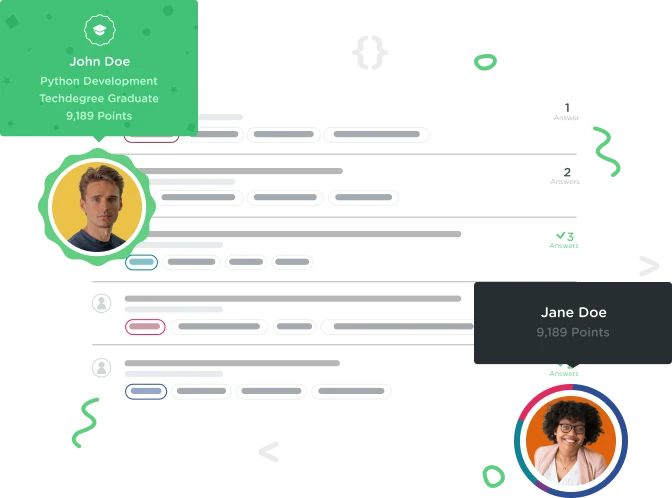

Casey Townsend
9,599 PointsWhen trying to check my work, I get an error stating that "The table should contain 6 rows and 6 columns."
I'm not sure where the requirement for having 6 rows and 6 columns came from. The instructions provide an example of a maxFactor of 3. I tried assigning 6 to the maxFactor parameter, and I'm still getting the same error when I recheck my work.
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[][] BuildMultiplicationTable(int maxFactor)
{
int[][] multiplicationTable = new int[maxFactor][];
for (int row = 0; row < maxFactor; row++){
multiplicationTable[row] = new int[maxFactor];
for (int col = 0; col < maxFactor; col++) {
multiplicationTable[row][col] = row*col;
}
}
return multiplicationTable;
}
}
}
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Casey,
Your dimensions are 1 less than they need to be. In the example, a maxFactor of 3 should produce a 4x4 array because you have that first row and column of all zeroes.
So in your code, you'd be creating arrays of size 3 but they need to be size 4 assuming 3 was passed in for maxFactor.
If you add 1 to maxFactor everywhere you're using it then that could be 1 way to get the right dimensions.
Or you could do something like
int tableSize = maxFactor + 1;
at the top of your method and then use tableSize everywhere you're using maxFactor. This way you don't have these + 1
everywhere.

Mike Wagner
23,559 PointsYou just need a maxFactor++;
at the top of your BuildMultiplicationTable()
method to extend the dimensions by one in each direction. It's a little misleading but, given the example, it shows a 4x4 for a maxFactor=3, like Jason Anello pointed out. I'm not entirely sure why the Challenge expects a +1 dimension rather than simply taking what it should. I assume because the "0" row/column is sort of useless information in a multiplication table program, but maybe I'm looking at it wrong.

Jason Anello
Courses Plus Student 94,610 PointsmaxFactor++
is going to change the value of maxFactor
. Throughout the rest of the method, maxFactor
won't have the same meaning as it did when it was first passed in.

Mike Wagner
23,559 PointsJason Anello - Wouldn't that essentially be the same as creating an entirely new variable and then using that instead of maxFactor throughout the method? Since the maxFactor
we're working with is internal to the method, as long as we're not redefining it on a scope that's larger than the method, and supposing we don't need the original value of maxFactor
for some other part of the method, I'm not sure what the difference is. I suppose in practice, if you wanted to extend the method it would require a slight refactoring, later down the line. If the method is as complete as it's going to get, though, I'm not seeing a difference. Perhaps I'm missing something. I'm only asking for clarification, not arguing against your logic.

Jason Anello
Courses Plus Student 94,610 PointsIt will be the same in terms of final result but different in terms of readability.
Generally we choose descriptive variable names to indicate what the variable is storing.
maxFactor
would indicate that it's storing the maximum factor.
In the case of the challenge example, it's 3.
When you increment it, it's now storing the size of the table which is 4. 4 is not the maximum factor. The variable name is no longer descriptive of what it's storing.
In my opinion this makes the code harder for others to read and even your future self.

Mike Wagner
23,559 PointsJason Anello - I would agree with that in some ways, I suppose. Though, I'm not sure it makes much of a readability difference in terms that maxFactor
is defined as 3 and returns a 4x4 table in the way that it is used. In some ways, it might even be argued that since a 4x4 table is expected, given the use described, that it actually makes the code more readable to increment maxFactor
to be the same size within the method, but I also see your point.
I'm just glad to know that I didn't miss some interesting C# voodoo edge-case and that it was, instead, more of a personal taste than anything else.