Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial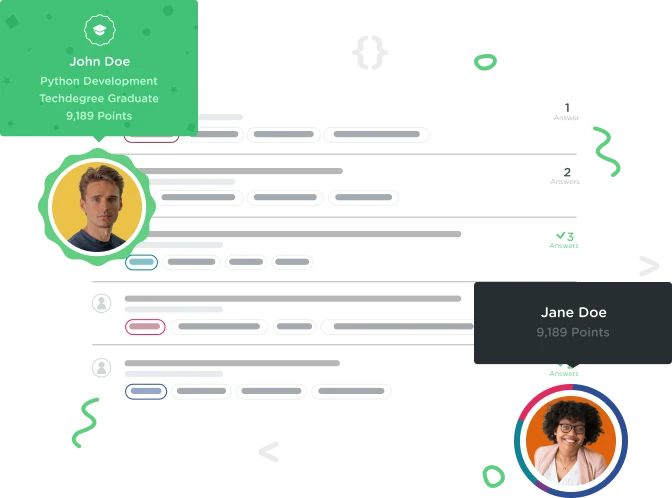

Pierce Mulligan
1,106 PointsWhen trying to eat the pez my program totally broke.
Really not sure what went wrong here, there were even errors where the code was previously working without any issues.
Example.java code:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making some funky Pez Dispensers.");
PezDispenser dispenser = new PezDispenser("Yoda");
System.out.printf("The dispenser character is %s\n",
dispenser.getCharacterName());
if (dispenser.isEmpty()) {
System.out.println("The dispenser is empty");
}
System.out.println("Loading Dispenser...");
dispenser.load();
if(!dispenser.isEmpty()) {
System.out.println("Dispenser isn't empty");
}
while(dispenser.dispense()) {
System.out.println("Chomp");
}
if(dispenser.isEmpty()) {
System.out.println("All the pez are gone!");
}
}
}
PezDispenser.java code:
public class PezDispenser { public static final int MAX_PEZ = 12; private String mCharacterName; private int mPezCount;
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
public boolean dispense() { boolean wasDispensed = false; if (!isEmpty()) { mPezCount--; wasDispensed = true; } return wasDispensed;
public boolean isEmpty() { return mPezCount == 0; } public void load() { mPezCount = MAX_PEZ; } public String getCharacterName() { return mCharacterName; } }
2 Answers

Christopher Stöckl
19,795 PointsHi Pierce!
You need to reformat your question, as the code is very hard to read at the moment :)
But I think I still managed to find the problem.
It seems like you are missing the last closing bracket after you return the "wasDispensed" variable.
It should look like this:
public boolean dispense() {
boolean wasDispensed = false;
if (!isEmpty()) {
mPezCount--;
wasDispensed = true;
}
return wasDispensed;
}
Hope that helps :)
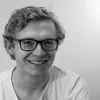
Luke Glazebrook
13,564 PointsHello Pierce!
Do you have any information about the error that you can tell us? With this additional information we will be able to solve your problem faster.