Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial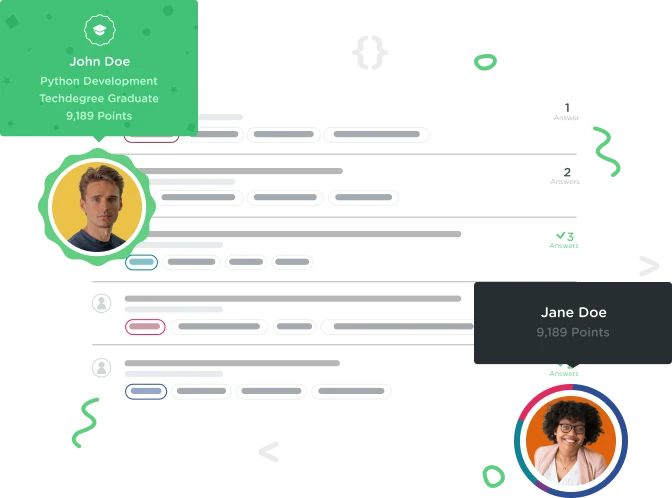

Cristi Roberts
5,905 PointsWhen trying to register my widget, it doesn't show up in the widget area.
In the video 'how to create a wordpress plugin', I'm stuck on the part where you register a widget. My widget doesn't show up in the widget area of my site. Here is my code: class Wptreehouse_Badges_Widget extends WP_Widget {
function wptreehouse_badges_widget() {
// Instantiate the parent object
parent::__construct( false, 'Official Treehouse Badges Widget' );
}
function widget( $args, $instance ) {
// Widget output
extract( $args );
$title = apply_filters( 'widget_title' , $instance['title'] );
$options = get_option( 'wptreehouse_badges' );
$wptreehouse_profile = $options['wptreehouse_profile'];
require( 'inc/front-end.php' );
}
function update( $new_instance, $old_instance ) {
// Save widget options
$instance = $old_instance;
$instance['title'] = strip_tags($new_instance['title']);
return $instance;
}
function form( $instance ) {
// Output admin widget options form
$title = esc_attr($instance['title']);
require( 'inc/widget-fields.php' );
}
}
function wptreehouse_badges_register_widgets() { register_widget( 'Wptreehouse_Badges_Widget' ); }
add_action( 'widgets_init', 'wptreehouse_badges_register_widgets' );
2 Answers
Henrik Hansen
23,176 PointsThe issue is how you name the constructor function. There are a couple of ways you can make this work:
<?php
class Your_Class_Name extends Parent_Class_Name {
// the constructor function, notice that the same letters are capitalized!
function Your_Class_Name () {
parent::__construct($arg1, $arg2);
//do init stuff
}
// other functions should have lower case letters:
function your_other_function() {
//do stuff
}
}
?>
The more preferred way should be to use __construct:
<?php
// Camel-case is preferred outside of wordpress:
class YourOtherClassName extends ParentClassName {
// This is much easier to understand:
function __construct() {
parent::__construct($arg1, $arg2);
//do init stuff
}
function otherFunction() {
}
}
?>
If you leave out the construct function, the parent __construct will automatically be called. If you want to include parameters when creating your object, just put some params like this:
<?php
class YourClass {
function __construct( $arg1, $arg2 ) {
//do stuff
}
}

Cristi Roberts
5,905 PointsThank you Henrik. That helps a lot!
Henrik Hansen
23,176 PointsI should add that the __construct() way is for php 5+ and the other is for php 4.