Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial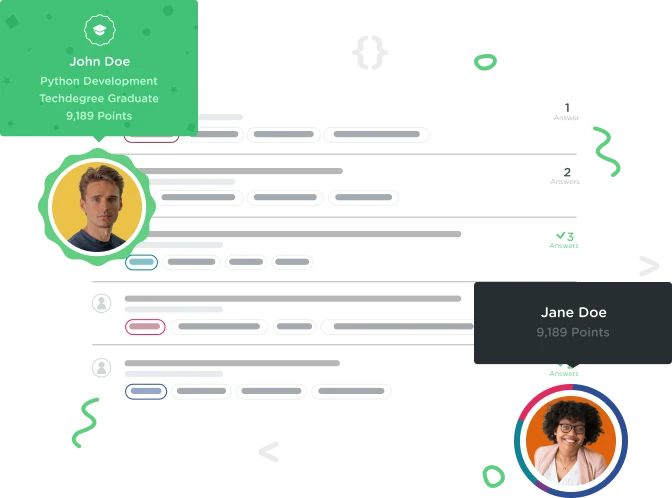
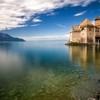
nakalkucing
12,964 PointsWhen trying to run D6 I keep on getting: AttributeError: type object 'D6' has no attribute 'value' Help please!
Here's my code:
import random
class Die:
def __init__(self, sides=2, value=0):
if not sides >= 2:
raise ValueError("Must have at least 2 sides")
if not isinstance(sides, int):
raise ValueError("Sides must be a whole number")
self.value = value or random.randint(1, sides)
class D6(Die):
def __init__(self, value=0):
super().__init__(sides=6, value=value)
I enter the Console and type:
cd yatzy/
python
import dice
d6 = dice.D6
d6.value
...and that's when I get the AttributeError.
Thanx in advance. :)
3 Answers
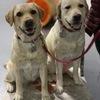
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Nakalkucing,
The issue is because you are trying to access the value
attribute on the class, not an instance of the class.
In your line:
d6 = dice.d6
You are assigning the d6 class to your d6
variable. If you wanted to assign an instance of d6 to your d6
variable you would add parentheses to instantiate the instance:
d6 = dice.d6()
Hope that clears things up
Cheers
Alex
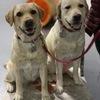
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Nakalkucing,
Your problem is in the Die class where you have two initialisers (which I think is syntactically invalid, but even if it is technically valid it is definitely not Pythonic).
When you try to create a D6 object, D6's initialiser doesn't know which initialiser in super() to call, and is looking at your second one and then getting upset because the second one doesn't take sides
as an argument.
The solution is just to delete the extraneous second initialiser.
Cheers
Alex
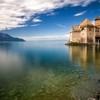
nakalkucing
12,964 PointsHi Alex, I'm not saying you're wrong, but I just cheked with the video and Kenneth's code has the second initialiser. Do you have any ideas to make it work without removing it? If not it's fine. :) Thanks.
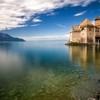
nakalkucing
12,964 PointsHello again. I found my mistake. :) I had written:
def __init__(self):
return self.value
It was supposed to read:
def __int__(self):
return self.value
Thanks for your time and help. :)
Best,
Nakal
nakalkucing
12,964 Pointsnakalkucing
12,964 PointsThanks Alex! That fixed the problem. :-D
nakalkucing
12,964 Pointsnakalkucing
12,964 PointsHi Alex Koumparos I'm now having more trouble with the code. I've done some of the changes in the next video. And I'm now getting this *line 36, in init super().init(sides=6, value=value) TypeError: init() got and unexpected keyword argument 'sides' * But I haven't changed that line. Here's my code. Would you please help me with it?