Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial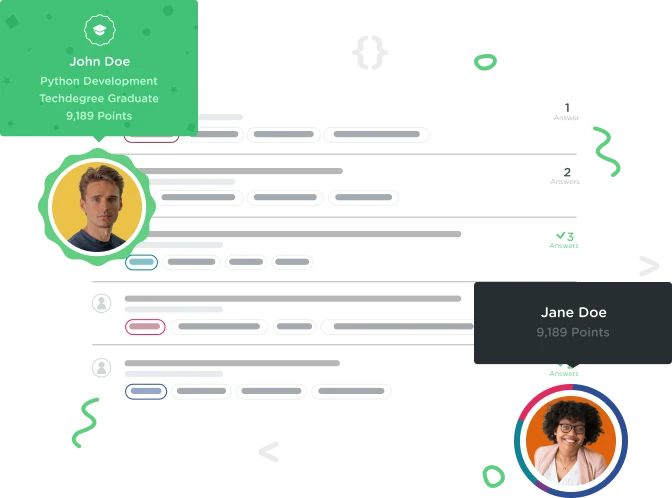

Tim Oltman
7,730 PointsWhen using an arrow function 'this' doesn't work inside the callback. Why?
When I set up the callback function as an arrow function I need to provide an event parameter and use event.target.style.display = 'none'. However, in the solution, they are able to use the 'this' keyword by defining the callback function as an anonymous function with no parameters. I'm just curious why it works this way.
To be specific, the following code results in: Uncaught TypeError: Cannot set property of 'display'
.
document.getElementById('begin-game').addEventListener('click', () => {
game.startGame();
this.style.display = 'none';
document.getElementById('play-area').style.opacity = '1';
})
However, only changing the function definition allows the code to work.
document.getElementById('begin-game').addEventListener('click', function() {
game.startGame();
this.style.display = 'none';
document.getElementById('play-area').style.opacity = '1';
})
3 Answers

Rich Donnellan
Treehouse Moderator 27,708 PointsArrow functions don't have their own bindings to this
, arguments
, super
, or new.target
keywords. Your second example is exactly what it takes if you do require any of these.
This article might help explain things better: https://www.codementor.io/@dariogarciamoya/understanding-this-in-javascript-with-arrow-functions-gcpjwfyuc

Bobby Verlaan
29,538 PointsPlease mind you can still use an arrow function. Instead of using this
, you can use the event object and select the button that was clicked on like this:
const btnStartGame = document.querySelector('button#begin-game');
btnStartGame.addEventListener('click', (e) => {
game.startGame();
e.target.style.display = 'none';
document.getElementById('play-area').style.opacity = '1';
});

Tim Oltman
7,730 PointsThanks for the reply. I thought that an arrow function and an anonymous function were completely equivalent.
Rich Donnellan
Treehouse Moderator 27,708 PointsRich Donnellan
Treehouse Moderator 27,708 PointsQuestion updated with code formatting. Check out the Markdown Cheatsheet below the Add an Answer submission for syntax examples.