Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial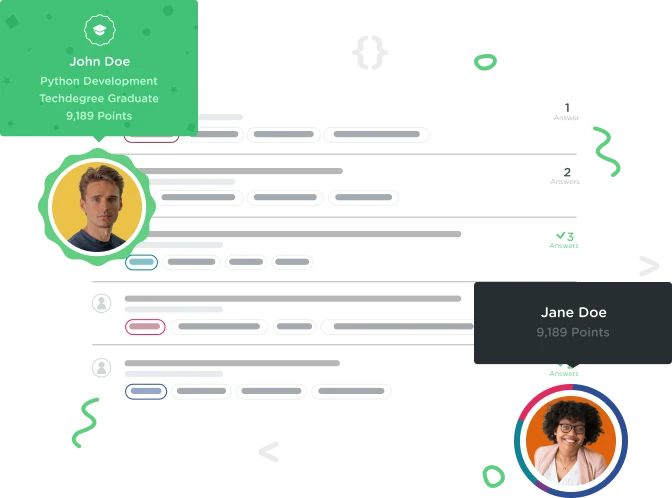
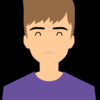
Dustin Joseph
2,898 PointsWhen Warrior is converted to a string, the string should be like "Warrior, <weapon>, <attack_limit>". Update your class
When Warrior is converted to a string, the string should be like "Warrior, <weapon>, <attack_limit>". Update your class to produce this string using values from the instance.
I am not sure what i am doing wrong here. I have looked all over the forums about this question but cant find anything. Any help is appreciated.
from character import Character
class Warrior(Character):
weapon = 'sword'
string = 'Warrior'
def rage(self):
self.attack_limit = 20
def print_warrior(self):
string = "{}, <{}>, <{}>".format(string, weapon, rage())
return string
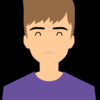
Dustin Joseph
2,898 PointsDavid McCarty Thanks for the response! So i replace the print_warrior with str, is that what you meant? It didn't seem to work for me. (I apoligize if i miss something, its late at night haha)
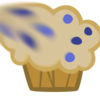
David McCarty
5,214 PointsYes, what you need to do is replace print_warrior with __str__
and have it just return the string that you have setup in your original code.
Just return the string like this
def __str__(self):
return "string {}".format(blah)
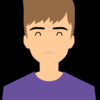
Dustin Joseph
2,898 PointsStill not working. This is my code: def str(self): return "string {}".format(Warrior, self.weapon, self.attack_limit)
Im thinking it is something with how i am ending the code ^^
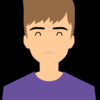
Dustin Joseph
2,898 PointsI got it now. Thank you so much! :)
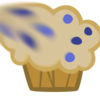
David McCarty
5,214 PointsYeah man, happy to help! Glad you got it working.
If you want, for others in the future, you can select my answer as the best answer so it's easier for others to see how you solves the problem :)
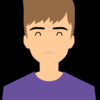
Dustin Joseph
2,898 PointsI would choose you as best answer if i actually knew how haha
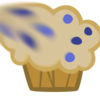
David McCarty
5,214 PointsI believe there should be a button on the bottom of my first comment that says "best answer" with a little check mark next to it. :)
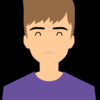
Dustin Joseph
2,898 PointsIts not there for me. I read up on it and it says that if you reply as a comment and not an answer i am not allowed to select you as best answer? maybe that's it?
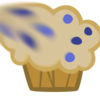
David McCarty
5,214 PointsHuh, that's odd.
Well, no worries! :)
Just glad I was able to help you figure out the problem!
Take it easy
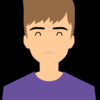
Dustin Joseph
2,898 PointsYeah thanks man. Have a good rest of your day :)
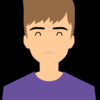
Dustin Joseph
2,898 PointsKen Alger Thank you!

Ken Alger
Treehouse TeacherThat's why the moderators are here.
2 Answers
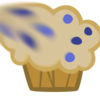
David McCarty
5,214 PointsThe problem is, you're not sticking with what they wanted you to have outputted.
You're doing:
def __str__(self):
return "string {}".format(Warrior, self.weapon, self.attack_limit)
And the problem is, you need it to come out as "Warrior, weapon, attack_limit"
All you really have to do at this point is change the string. Also, you don't need to put Warrior in the .format arguments, you just need the weapon and the attack_limit for two separate placeholders

Ken Alger
Treehouse TeacherChanged this comment to an answer
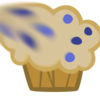
David McCarty
5,214 PointsOh cool! Thanks man!

Peter Rzepka
4,118 Pointsfrom character import Character
class Warrior(Character): weapon = 'sword'
def rage(self):
self.attack_limit = 20
def __str__(self):
return "Warrior, {}, {}".format(self.weapon, self.attack_limit)
David McCarty
5,214 PointsDavid McCarty
5,214 PointsMy guess is that you're supposed to override the
__str__
method that returns a string.Basically, do what you're doing just make the method
__str__
and return the string instead of printing something.__str__
is what gets called whenever you try and print out an object. If there's nothing there, it basically just prints out the object name and the address in memory. What you're doing when you override the method is making the program call your special__str__
method whenever someone tries to print the object.