Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial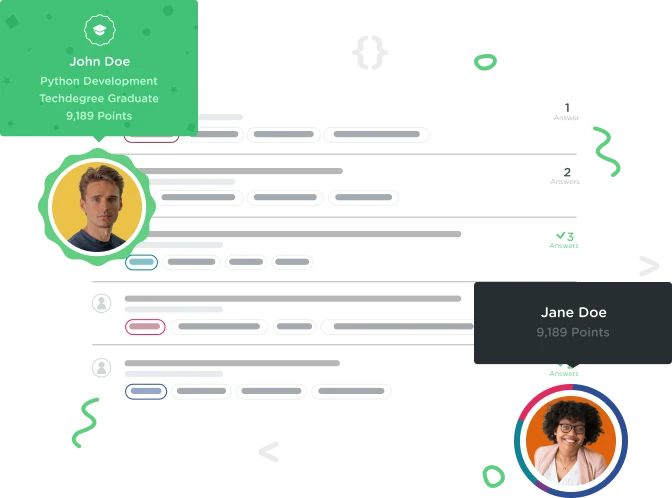

Chris Galatis
3,089 PointsWhen we give a method an argument, what is the argument actually doing?
I am a bit confused as to what the argument is used for when we define a method
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def contains?(name)
end
def find_index(name)
index = 0
found = false
todo_items.each do |item|
found = true if item.name == name
break if found
index += 1
end
if found
return index
else
return nil
end
end
end
2 Answers
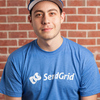
Dylan Shine
17,565 PointsWhen we provide an argument to a method in Ruby, we are creating a local variable for our method to use/operate on the method object we provided.
def initialize(name)
@name = name
@todo_items = []
end
For the standard initialize method, we provide an argument called name, probably in the form of a String, that we will use to assign the instance variable @name. We are simply passing a variable or value to that method, and the method will do something with or to it.
my_num = 1
def do_something_to_num(number)
number *= 2
end
do_something_to_num(my_num) #=> 2
Here we create a variable my_num, and define a method do_something_to_num that takes one argument, number. Second we invoke the our newly defined method, passing in the variable my_num for it's argument.
To answer your question, arguments are representing/providing values for our methods, so your methods can perform whatever their trying to do.
Best,
Dylan

Chris Galatis
3,089 Pointsso if i am understanding it right the arguments we give the methods are kind of like characteristics of the method? like the argument is what the method does? as in this code
my_num = 1 def do_something_to_num(number) number *= 2 end
do_something_to_num(my_num) #=> 2
so the do_something_to_num method is taking an argument (number) and that arguments multiples by 2. so when the do_something_to_num method is called in the second part, it takes the my_num variable that we set equal to 1 and *= 2 onto my_num if i am understanding correctly?
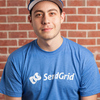
Dylan Shine
17,565 PointsCorrect