Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial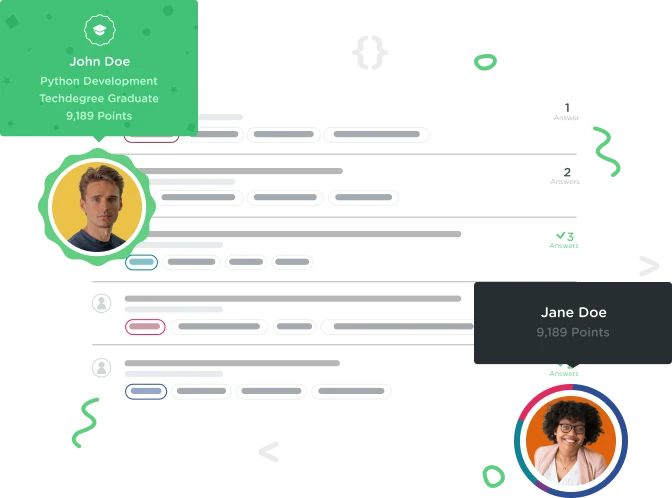

Isaac Wong
2,106 PointsWhen we initialize a subclass, we first need to initialize the properties in our base class, then call the super class'
One of the quiz questions in the section "Build a Simple iPhone App...": When we initialize a subclass, we first need to initialize the properties in our base class, then call the super class' initializer
True False
The answer is True but it seems to contradict the video "Overriding Properties" at 1:24: https://teamtreehouse.com/library/objectoriented-swift-20/class-inheritance/overriding-properties
Initializing a subclass
- Provide values for properties of the subclass
- Once the subclass is initialized, provide values for the properties of the base class
I could be interpreting this incorrectly. Any thoughts?
1 Answer
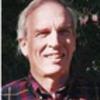
jcorum
71,830 PointsUnless I'm really missing the point, it seems that "initializing the properties in our base [super] class" and "calling the super class' initializer" amount to the same thing. The way you initialize the properties of the base class from the subclass is to call the super class's initializer. So saying we do one and then the other is really misleading.
But they may have meant "initializing the properties in our sub class", and if so, then true is the right answer.
There's an example in the Swift documentation for class inheritance and initialization, but the subclass doesn't have any properties of its own. So I took the liberty of adding one (airpump):
class Vehicle {
var numberOfWheels = 0
var description: String {
return "\(numberOfWheels) wheel(s)"
}
}
class Bicycle: Vehicle {
var airpump: Bool
override init() {
airpump = true
super.init()
numberOfWheels = 2
}
}
If you try to call super,init() before initializing airpump you will get an error.
The point of the example in the documentation is that you cannot modify a superclass property (numberOfWheels) before you have called the superclass's initializer and let it do its job first.