Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial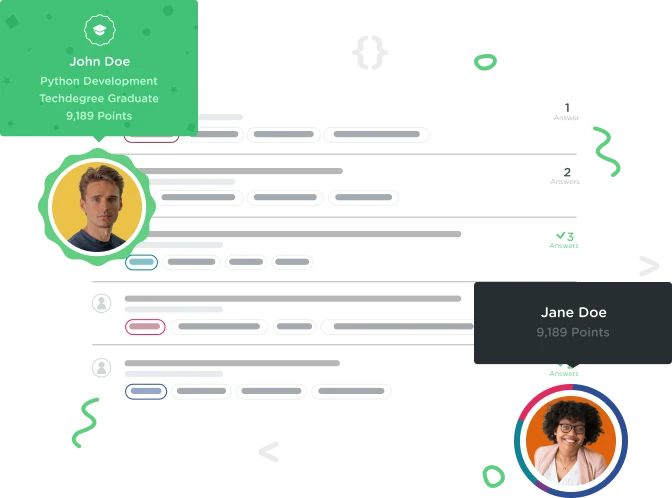

Ryan Wikle
5,774 PointsWhen would it be a good practice to use a switch instead of an if else statement? Also what are its advantages.
When would it be a good practice to use a switch instead of an if else statement? Also what are the advantages of using the switch method?
1 Answer

Indiya Gooch
12,075 PointsTL/DR: Advantages:
- Can help keep code DRY(don't repeat yourself)
- Easier to read over long if else if statements
- Easier to maintain over long if else if statements
Usage: If you find yourself building an if else if statement and you've got more than 2 conditions on top of the initial if() condition, you should consider making it into a switch statement instead.
Full Explanation: Similar to the If statement, the switch statement is used for multiple branching logic.
switch (expression) { case value1:
//code to execute when the result of expression matches value1 break;
case value2:
//code to execute when the result of expression matches value2 break;
}
The parentheses contain the condition or expression to be evaluated against each case clause. You can have as many cases as you want to test against the expression, and if the expression matches a specified value in a case, the statements inside the matching case clause are executed until either the end of the switch statement or a break.
Break statements are highly recommended as they tell the JavaScript interpreter to jump out of the switch statement once they reach them, and continue on in the program, if the break is missing, the statement will fall through to the next code (case) even if the evaluation does not match the case causing unwanted behaviours.
The switch statement also provides a default option that acts in a similar way to an an else block in an if else statement.
let goneOut = prompt('Has the dog been walked? Yes or no'); switch (goneOut) {
}
switch(goneOut) {
case 'yes':
console.log( "Dog has been walked!"); break;
case 'no':
console.log( "The dog needs walking!"); break;
default:
console.log( "Answer not understood!");
}
Sometimes you will want different switch cases to execute the same code if their value matches the expression, instead of repeating the code you can keep it DRY by stacking the cases.
If multiple cases match the expression, the first case is selected. If no matching cases are found, the program continues to the default label, and if this is not found it will continue to the statements after the switch.
Note: switch uses the identical comparison operator to compare cases to the expression, so be mindful that the expression or cases may need to be changed to comply with case sensitivity and data type.
switch (goneOut.toLowerCase()) { case 'yes':
console.log( "Dog has been walked!"); break;
...
}