Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial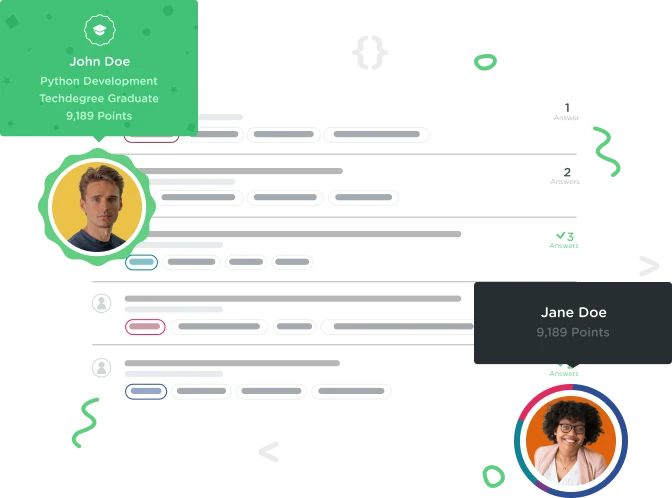

Derick Brown
7,361 PointsWhen would we use Arrow Function Syntax?
So when would we use Arrow Function Syntax? Only when we're assigning a function to a variable? (in this case, a "const" since it doesn't change)
3 Answers
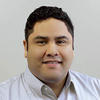
miguelorange
1,772 PointsI'd say wherever you are used to using a function.
Map/Filter
const numbers = [1,2,3,4];
const numbersSquared = numbers.map(number => number * numbers));
that's not to say you have to assign it to a variable.
console.log('Number One', numbers.filter(number => number == 1));
React
const MessageComponent = props => <div><h1>Hello, {props.name}</h1></div>;
const MessageComponent = ({name}) => <div><h1>Hello, {name}</h1></div>;
Function without variables
const helloMessage = () => console.log('Hello!');

Jones Dias
13,902 PointsAll cool examples! Btw, you should never use it when you need to use the this
keyword in your function. Arrow functions doesn't work with them. Check it out
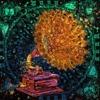
jaspergradussen
5,876 PointsThanks, I wondered about this!

Sarai Vasquez Mariñez
3,891 PointsThat was really useful! Many thanks

Michelle Cannito
8,992 PointsThe tutorial mentioned variable scope as a reason to use arrow functions, but unfortunately failed to explain. This link has a good explanation: http://pointdeveloper.com/es6-for-beginners-es6-arrow-functions-and-scope/ In that example, the "this" keyword gives an "undefined" error when the function is written without the arrow. You can search for "arrow functions and scope" for more information.
There are also times when NOT to use an arrow function, and here is a link: https://rainsoft.io/when-not-to-use-arrow-functions-in-javascript/ It basically states that if you want to call a method in an object, you need a name to call.
I just reactivated my TeamTreehouse membership today after several years absence, and this mini presentation is the first I watched. I'm disappointed that the "why" (scope) is not covered, even though mentioned, only the how. Hoping the other courses, particularly Angular, do not have gaps in basic information...
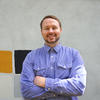
Tom Geraghty
24,174 PointsHey Michelle, just wanted to drop a quick comment in about why Treehouse isn't explaining the "why" in this video. This video is the fourth video in the Welcome to Beginner JavaScript track and as such Treehouse expects people who sign up for this track to be very beginner/new to programming. It's likely they didn't want to overwhelm and confuse people who have only had maybe 30 minutes in the previous few videos to get an introduction into programming concepts.
I understand where you're coming from; it'd be nice if there were different ability levels for each video so they can spend time going into details about scope and binding this for those of us who want to know that info. However here I think this video is just to let you know that arrow functions exist and what their syntax is, and you'll get the why, when to and when-not-to use them in later videos and courses (e.g.: the React track talks a fair amount about this binding when calling functions on and within components).
Derick Brown
7,361 PointsDerick Brown
7,361 PointsThanks for the information! It just sucks that I spent hours refreshening up on my Javascript to learn everything I learned is obsolete hahaha. But I'll adjust. Just need to study it a bit more and get used to it.
So basically, don't use this anymore...
but instead use this (if I typed it correctly)...
const variableName = funcName = () => (whatever else goes after. I'm not used to this format yet lol)
correct?
miguelorange
1,772 Pointsmiguelorange
1,772 PointsDerick Brown , Close You have too many equal signs and I'm not sure if you intended to use () surrounding whatever else goes here.... When adding arrow function to your utility belt take it step by step. Get comfortable changing function to const ,and where to throw in the arrow:
^^ not that much different. Then understand when to refactor. Is the code a one liner - remove (), {}, and move to one line.
const funcName = name => // do something
Agreed seems like sugar. But you have net results when you consider the cool features of rest and destructuring Good Luck