Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial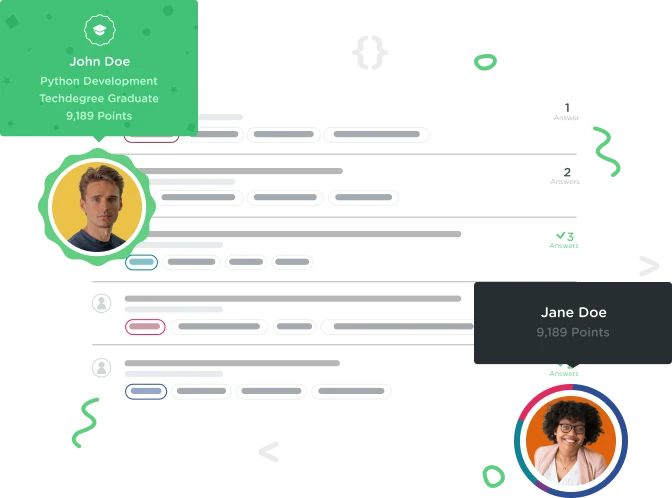

mk37
10,271 PointsWhen you finally create the real data/function, do you remove the stubs/mock/fake data afterwards?
I do not think this was made clear and I guess I am still wrapping my head around with unit testing. But after the real codes are done for unit testing, would it make sense to get rid of the fake data?
2 Answers
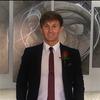
Liam Clarke
19,938 PointsHello
You use the same function for both your test and your mockdata, you just import/require that function into the test folder to use it.
Ill use your example above:
// ./main.js - you actual file
function functionOne (para1) {
return para1;
};
// .main.spec.js - your test file
describe('A suite is just a function', function() {
// Before each spec we want to require the file we are testing
beforeEach(function() {
const component = require('./main.js');
});
// Within the test we get the main.js function from the actual file
it("and so is a spec", function() {
expect(component.functionOne(2)).to.equal(2);
});
});
As you can see i want to test the actual function but we provide "mock data" by the number of 2 to see if the actual function returns the correct value.
Hope this helps!
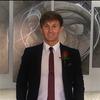
Liam Clarke
19,938 PointsHello
You should always keep your mock data, this should also always simulate your real data. Its good for keeping a history of what you tested.
This is just used to test functionality without making costly requests to real data.
For example: lets say a snippet of your real database looked like this, you want to copy that and test it works as intended with mock data
[
{
"id": "0001",
"name": "John Doe",
},
{
"id": "0002",
"name": "Jane Doe",
}
]
You want to simulate the real database with your mock one but as far as your test is concerned, you just care about what data is returned, your test is just ensuring the functionality happens.
So a test for a function
getsUserById(0002); // return 1 object from the mock data
Our test would check the functionality works to return 1 user with that same ID.
Or maybe you want to test that it will return an error when a ID doesn't exist:
getUserById(1234); // return error
So your tests are just a way of you using some data without using the real thing, allowing you to test any outcome whether that is a good request, 400 bad request, 401 unauthorized, 404 not found, 408 timeout... the list goes on and you can change the mock data to test each and every outcome.

mk37
10,271 PointsThanks for the reply, much appreciated.I get what you are saying, but I guess what the confusing part for me is, when you create the mock and real data that has the same name declared, would that not cause conflict and confusion for the test. How would the test know which one to test for. For example:
//test file
function functionOne (para1) {
return para1;
};
expect( functionOne(2) ).to.equal(2);
Than when you finally come round to creating the real function on you main code file and export it to the test file:
//main js file
function functionOne (para1) {
return para1;
};
It would give error saying functionOne is already declared.
I understand this is a very simple example, and you could write functionOne on the main js file to begin with. But what about very complicated data (eg. ajax) that you want to create on the fly so you can test with. Surely you would need to eliminate them afterwards if they have the same declared name.
Perhaps I am overthinking it or not getting it, but unit testing has giving me the most headache, especially with the inclusion of sinon.js. It just seem testing was design for backend developers first, and frontend is an afterthought.