Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial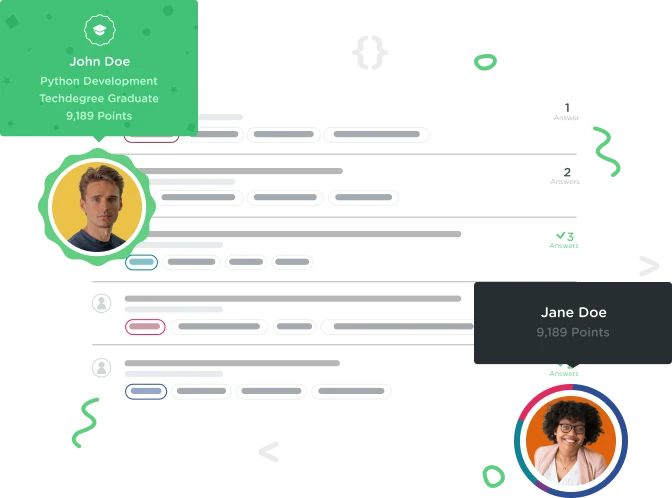
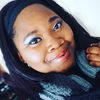
kalina edwards
5,454 PointsWhenever I type 'list' it isn't displaying anything to the screen.
Whenever I type 'list' it isn't displaying anything to the screen. Sometimes when I type 'quit' it will display the full list or it will end the program and do nothing.
Here is the code:
var inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese',
'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato',
'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write( '<p>' + message + '</p>');
}
while ( true ) {
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit");
if ( search === 'quit') {
break;
} else if (search === 'list') {
print(inStock.join(', '));
}
}
5 Answers
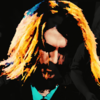
Justin Horner
Treehouse Guest TeacherHello Kalina,
I think what you're experiencing is a change in the rendering priority the browser uses to decide when to render page content and display the prompt dialog. In the latest version of Chrome and Safari, the list will not render on the page until the dialog stops displaying.
Here's an alternative approach that drops the while loop in favor of recursion so that we can use setTimeout to call the showPrompt function again. It's the use of the setTimeout function that allows the UI to render our changes to the page before calling prompt again.
Search.js
var inStock = ['apples', 'eggs', 'milk', 'cookies', 'cheese',
'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato',
'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write('<p>' + message + '</p>');
}
function showPrompt() {
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit");
if (search === 'quit') {
return;
} else if (search === 'list') {
print(inStock.join(', '));
setTimeout(showPrompt, 0);
}
}
index.html
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body onload="showPrompt()">
<script type="text/javascript" src="search.js"></script>
</body>
</html>
I hope this helps.
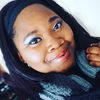
kalina edwards
5,454 Pointsvar inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese',
'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato',
'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write( '<p>' + message + '</p>');
}
while ( true ) {
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit");
if ( search === 'quit') {
break;
} else if (search === 'list') {
print(inStock.join(', '));
}
}
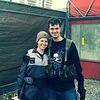
Christopher Parke
21,978 PointsJust quickly guessing but maybe it's because you're using strictly equals "===" instead of evaluates to "==".
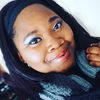
kalina edwards
5,454 PointsThanks but it seems to be okay. I just realized that when I type the word 'list' the loop keeps going until I type in quit. It doesn't print my list until after the word 'quit' is typed. That is the issue I am having because in the video lecture, Dave types 'list' and it automatically prints, But none of my things list until after I type quit. Weird. Still need some help with that if anyone has any suggestions.
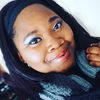
kalina edwards
5,454 PointsThank you for the help. I will look up more on the DOM with those restrictions. You've been very helpful.

Tommy Leng
Front End Web Development Techdegree Student 12,417 PointsYou could type in "break" after " print(inStock.join(','));". Then it gets out of the while loop and displays it on the browser as well.
kalina edwards
5,454 Pointskalina edwards
5,454 PointsThank you for your reply I checked it out and it works. Thank you so much. The only thing is I wish I could use the while loop to do it. :)
Justin Horner
Treehouse Guest TeacherJustin Horner
Treehouse Guest TeacherYou're welcome, kalina edwards! Yes, it's unfortunate that all the browsers do not implement their functionality in the same way. Of course, nothing is wrong with sticking to the while loop. Although it doesn't work as expected in Chrome and Safari, it does work in Firefox.
The important take away here is that there's no fault in your code. It's how Chrome and Safari handle DOM changes in a tight loop that continuously displays a prompt dialog.
Happy coding :)