Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial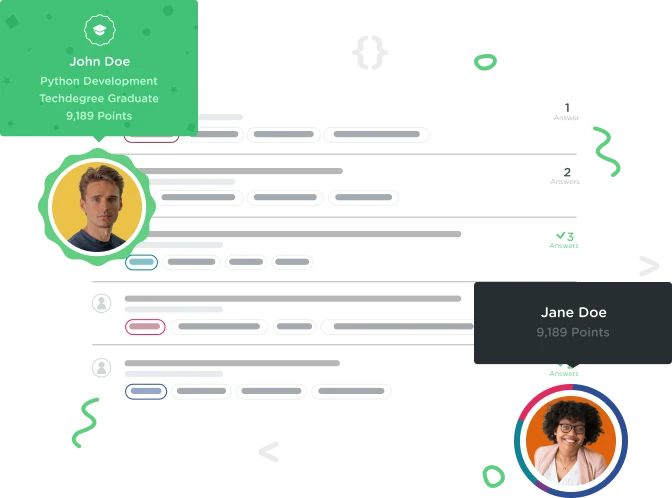

Andrew Stevens
11,083 PointsWhere am I going wrong?
For the life of me I can't figure out why I am receiving error cannot find symbol Method[].
Please see my code attached, where am I going wrong and what am I failing to understand here?
public class TestAnalyzer {
/**
* Counts the number of methods in the class given by `clazz` that have been annotated
* with the @Test annotation.
*/
public static int getNumAnnotatedMethods(Class<?> clazz) {
int count = 0;
Method[] method = clazz.getDeclaredMethods();
for (Method methods : method) {
if (methods.annotationIsPresent()) {
count = count + 1;
}
}
return count;
}
}
2 Answers
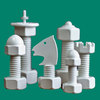
Steven Parker
231,275 PointsDid you notice the message when you ran the test? It contains a hint:
Bummer: Oops, there is a compiler error! Don't forget to add import statements for any outside classes you're using.
So try adding import java.lang.reflect.Method;
at the top.
Also, there's a couple other issues:
- instead of "annotationIsPresent", did you mean to write "isAnnotationPresent"?
- isAnnotationPresent requires an argument

eotfofiw
Python Development Techdegree Student 824 PointsThere are several reasons for this error message. In this particular instance, it means that Method[]
is referring to the Method
class. Given the code example above, the compiler doesn't know what that is.
There are some Java classes that are just available to you in the standard packages. Method
is not one of them. You will need to tell the compiler that in this file, you want to use classes that are found in the reflect package:
import java.lang.reflect.*;
With that statement at the top of your file, Java will know that everything (that's what the * does) in the reflect package should be available to code in this file. If you receive this error again, you can look up in the Java lang docs in which package you can find this class.
A lot of IDEs will also give you a list of possible packages you could import that contain a class that matches what you're asking to use and allow you to import them.