Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial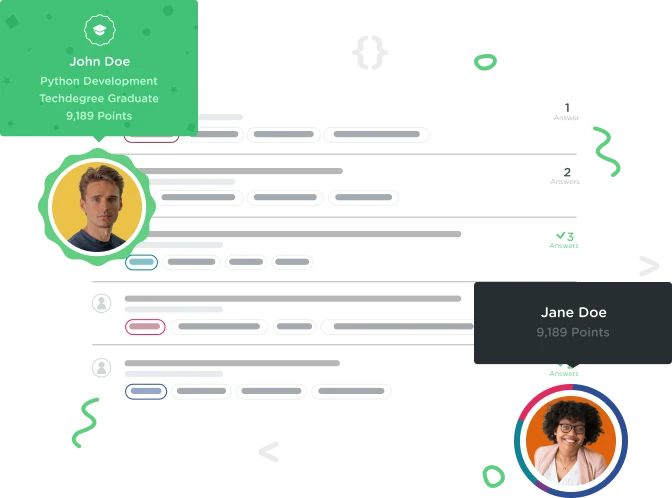

Atul Varma
4,285 PointsWhere am I going wrong with the code ?
You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(a):
try:
a = int()
return(a * a)
except ValueError:
a = str()
#print("It's a string")
return(a * len(a))
squared('a')
2 Answers

Samuel Ferree
31,722 Pointsyou need to pass a into the int and str functions, like so
a = int(a)
a = str(a)
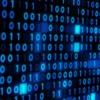
Alexander Davison
65,469 PointsAlso, to add onto Samuel Ferree's post, you should't call the squared
function.
The code challenge does this automatically for you :)
Good luck!
~Alex
Atul Varma
4,285 PointsAtul Varma
4,285 PointsIs it because we are using an argument when we define our function, we should use
a = int(a) a = str(a)
??
Samuel Ferree
31,722 PointsSamuel Ferree
31,722 Pointsint and str are functions that take variables as parameters, and they return those variables as integer and string types.
when using them to convert variables to different types, you always need to pass the variable into those functions.
Atul Varma
4,285 PointsAtul Varma
4,285 PointsThank You Soooo Much.
Atul Varma
4,285 PointsAtul Varma
4,285 Pointsdef squared(a):
squared('a')
Please help me out here--
If its an integer, it prints its square but if I enter any string, its not giving right output. I want to get o/p as following for any string I enter--
squared("tim") should return "timtimtim" ( argument multiplied by its length.)
Samuel Ferree
31,722 PointsSamuel Ferree
31,722 PointsYou don't need to input a, as it's passed in to your function squared.
If you try to convert the input to an integer as soon as you get it, and it fails, you never successfully store the input value in a, so when you try to convert it to a string later, there is nothing in it.