Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial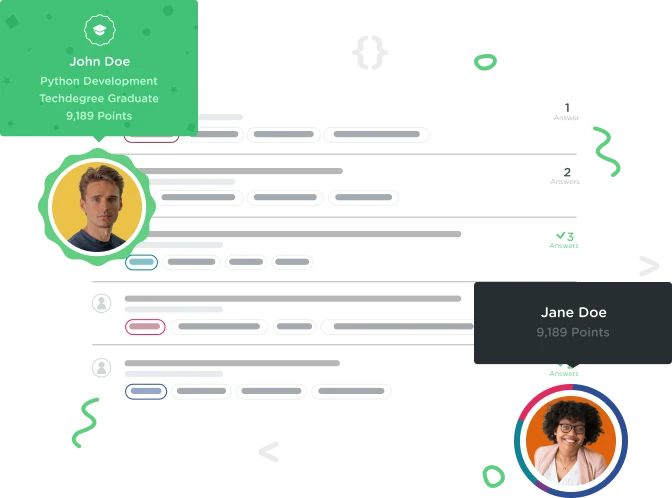
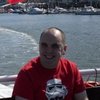
Sean Flanagan
33,235 PointsWhere are the errors?
Hi there.
I've got roughly as far as 1 m 15 s and already there are errors.
Example.java:
import java.util.Arrays;
import java.util.Date;
import com.teamtreehouse.Treet;
public class Example {
public static void main(String []args) {
Treet treet = new Treet(
"craigsdennis",
"Feeling overwhelmed by learning to #code?" +
"Try out this thought hack I whipped up: http://buff.ly/1LjAZrJ #development #webdev",
new Date(1437084178)
);
Treet treet = new Treet(
"journeytocode",
"@treehouse makes learning Java sooooo fun! #treet",
new Date(1421878767000L)
);
System.out.printf("This is a new Treet: %s %n", treet);
System.out.println("The words are:");
for (String word: treet.getWords()) {
System.out.println(word);
}
Treet[] treets = {treet, secondTreet};
Arrays.sort(treets);
}
}
Treet.java:
package com.teamtreehouse;
import java.util.Date;
public class Treet implements Comparable {
private String mAuthor;
private String mDescription;
private Date mCreationDate;
public Treet(String author, String description, Date creationDate) {
mAuthor = author;
mDescription = description;
mCreationDate = creationDate;
}
@Override
public String toString() {
return "Treet: \"" + mDescription + "\" - @" + mAuthor;
}
public String getAuthor() {
return mAuthor;
}
public String getDescription() {
return mDescription;
}
public Date getCreationDate() {
return mCreationDate;
}
public String[] getWords {
return mDescription.toLowerCase().split("[^\\w#@']+");
}
}
I've forked my workspace and I'll take a snapshot.
I'd appreciate any help.
Sean :-)
4 Answers
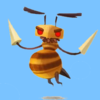
Tugrul Can Sollu
2,437 PointsHi Sean, Everything is good but there is a error at Example.java on line 25. You have renamed the 15'th line "secondtreet", it should be camel-cased( such as "secondTreet"). I'm afraid but java is really mad at "case sensitivity " things like that.
After all, I think you're ready to keep going! I couldn't answer your answer(so, i snet it as a another answer). I'm new in Treehouse forum. And sorry for my English.
Have good day!
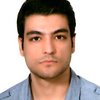
Sina Maleki
6,135 PointsHi Sean Flanagan, You didn't write the "compareTo" method out. you must to implement the "Comparable" method.
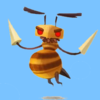
Tugrul Can Sollu
2,437 PointsWhat is the eror saying?
I think the problem is the 'L' in date.
In your code; Example.java
Treet treet = new Treet(
"craigsdennis",
"Feeling overwhelmed by learning to #code?" +
"Try out this thought hack I whipped up: http://buff.ly/1LjAZrJ #development #webdev",
new Date(1437084178)
);
I think this line
"new Date(1437084178)"
should be like below. ( watch out for 'L' )
new Date(1437084178L)
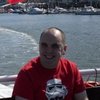
Sean Flanagan
33,235 PointsHi. Thanks for your reply. Here are the error messages:
./com/teamtreehouse/Treet.java:32: error: ';' expected
public String[] getWords {
^
Example.java:15: error: variable treet is already defined in method main(String[])
Treet treet = new Treet(
^
Example.java:22: error: cannot find symbol
for (String word: treet.getWords()) {
^
symbol: method getWords()
location: variable treet of type Treet
Example.java:25: error: cannot find symbol
Treet[] treets = {treet, secondTreet};
^
symbol: variable secondTreet
location: class Example
./com/teamtreehouse/Treet.java:5: error: Treet is not abstract and does not override abstract met
hod compareTo(Object) in Comparable
public class Treet implements Comparable {
^
./com/teamtreehouse/Treet.java:33: error: return outside method
return mDescription.toLowerCase().split("[^\w#@']+");
^
6 errors
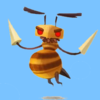
Tugrul Can Sollu
2,437 PointsHello Sean, I have review your code. Firstly, I should say you're doing great!
I found that you forgot some parenthesis at Treet.java on line 32. So compiler didn't understand what getWords is. Because of a wrong definition of getWords() method, there is another error occured at Example.java on line 25. It couldn't know what is getWord.
There is an another thing that occures error. In Example.java, you declared two Treet object and they have same names ("treet"). You should change the second Treet object's name (second Treet obejct is at 15'th line). In the course, teacher named it "secondTreet".
In Example.java on 25'th line, you are making a new Treet array. It will keep two Treet objects, because of wrong naming of secondTreet(It was "treet") compiler can not find a Treet object named "secondTreet". We have solved this problem by renaming Treet obejct at line 15.
After all this,there is one error left. It's about overriding an method of Comparable interface. You will learn how to override it in course videos . So i wont solve it. You should take the lessons and learn how to override. Believe me , it's really easy.
Here are the codes ( Sorry, if ı couldn't highligth them)
Example.java
import java.util.Arrays;
import java.util.Date;
import com.teamtreehouse.Treet;
public class Example {
public static void main(String []args) {
Treet treet = new Treet(
"craigsdennis",
"Feeling overwhelmed by learning to #code?" +
"Try out this thought hack I whipped up: http://buff.ly/1LjAZrJ #development #webdev",
new Date(1437084178L)
);
Treet secondTreet = new Treet(
"journeytocode",
"@treehouse makes learning Java sooooo fun! #treet",
new Date(1421878767000L)
);
System.out.printf("This is a new Treet: %s %n", treet);
System.out.println("The words are:");
for (String word: treet.getWords()) {
System.out.println(word);
}
Treet[] treets = {treet, secondTreet};
Arrays.sort(treets);
}
}```
Treet.java
```java
package com.teamtreehouse;
import java.util.Date;
public class Treet implements Comparable {
private String mAuthor;
private String mDescription;
private Date mCreationDate;
public Treet(String author, String description, Date creationDate) {
mAuthor = author;
mDescription = description;
mCreationDate = creationDate;
}
@Override
public String toString() {
return "Treet: \"" + mDescription + "\" - @" + mAuthor;
}
public String getAuthor() {
return mAuthor;
}
public String getDescription() {
return mDescription;
}
public Date getCreationDate() {
return mCreationDate;
}
public String[] getWords() {
return mDescription.toLowerCase().split("[^\\w#@']+");
}
}```
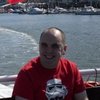
Sean Flanagan
33,235 PointsHi. Thanks for your help. I've given this another shot. Here's what it looks like now.
Example.java:
import java.util.Arrays;
import java.util.Date;
import com.teamtreehouse.Treet;
public class Example {
public static void main(String []args) {
Treet treet = new Treet(
"craigsdennis",
"Feeling overwhelmed by learning to #code?" +
"Try out this thought hack I whipped up: http://buff.ly/1LjAZrJ #development #webdev",
new Date(1437084178L)
);
Treet secondtreet = new Treet(
"journeytocode",
"@treehouse makes learning Java sooooo fun! #treet",
new Date(1421878767000L)
);
System.out.printf("This is a new Treet: %s %n", treet);
System.out.println("The words are:");
for (String word: treet.getWords()) {
System.out.println(word);
}
Treet[] treets = {treet, secondTreet};
Arrays.sort(treets);
}
}
Treet.java:
package com.teamtreehouse;
import java.util.Date;
public class Treet implements Comparable {
private String mAuthor;
private String mDescription;
private Date mCreationDate;
public Treet(String author, String description, Date creationDate) {
mAuthor = author;
mDescription = description;
mCreationDate = creationDate;
}
@Override
public String toString() {
return "Treet: \"" + mDescription + "\" - @" + mAuthor;
}
public String getAuthor() {
return mAuthor;
}
public String getDescription() {
return mDescription;
}
public Date getCreationDate() {
return mCreationDate;
}
public String[] getWords() {
return mDescription.toLowerCase().split("[^\\w#@']+");
}
}
Have I got it right? Thanks :-)
PS I've not watched the whole of the Interfaces video.
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsI've changed the 15th line again:
There's only one error now, in the comparable line, but I think I can fix it.
Thank you :-)