Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial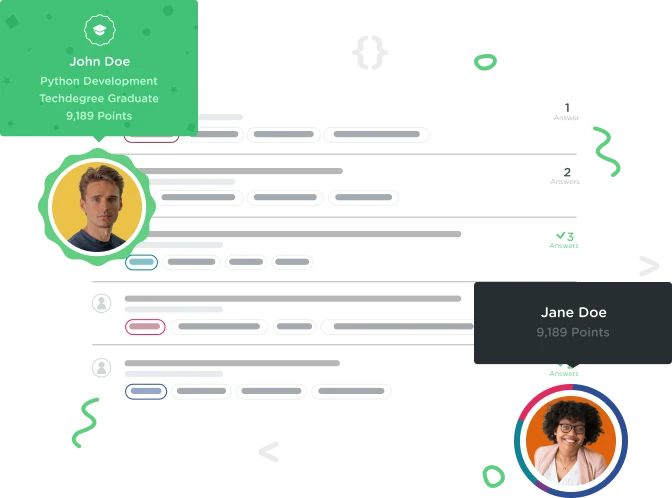

Roberto Correale
1,539 PointsWhere can i find 'babel-browser.min.js' on Babel's site?
Hi, I've got a question about Babel. In the vendor folder in the workspace, there is 'babel-browser.min.js', but i can't find it on babel's site.
Can you explain me the right way to download/import it in my project? (i know i can copy it from workspace, but i want to learn how to do that in the 'official way' in case of future updates of babel).
Thank you
4 Answers

Philip Howley
11,796 PointsIn order to have this work on my machine I did the following:
I used npm and here's my package.json
{
"name": "first-react-app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"react": "^15.3.1",
"react-dom": "^15.3.1"
},
"devDependencies": {
"babel-browser": "^6.1.19"
}
}
here's my html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Scoreboard</title>
<link rel="stylesheet" href="./app.css" />
</head>
<body>
<div id="container">Loading...</div>
<script src="./node_modules/react/dist/react.min.js" charset="utf-8"></script>
<script src="./node_modules/react-dom/dist/react-dom.min.js" charset="utf-8"></script>
<script src="./node_modules/babel-browser/browser.min.js" charset="utf-8"></script>
<script type="text/babel" src="./app.jsx"></script>
</body>
</html>
And here is my app.jsx
function Application() {
return (
<div>
<h1>Hello from React</h1>
<p>
I was rendered from the Application compontent!
</p>
</div>
);
}
ReactDOM.render(<Application/>, document.getElementById('container'));
Once all my files were ready, I installed http-server and ran it with $ http-server
and then I browsed to http://127.0.0.1:8080
and my code worked

Roberto Correale
1,539 PointsThank you Jason.
I was getting errors this way, trying the same code of workspace in my local machine. Maybe a good starting point for this course could be how to config React without babel-browser included directly in the project, considering that is not supported anymore.
Anyway thank you again Jason!

Gabriel Maajid
Courses Plus Student 1,545 PointsAgreed I ran into this problem as well. Looks like this needs course updating.

jmmarco
1,449 PointsYou can find the Babel standalone project here.
Here are the CDN's:
If you're trying to work on this project from local project folder on your computer. Then your index.html
should look something like this:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Intro React</title>
</head>
<body>
<div id="app">Loading...</div>
<script src="https://unpkg.com/react@15/dist/react.js"></script>
<script src="https://unpkg.com/react-dom@15/dist/react-dom.js"></script>
<script src="https://unpkg.com/babel-standalone@6/babel.min.js"></script>
<script type="text/babel" src="js/App.js"></script>
</body>
</html>
Note: I did not include any stylesheet for my project. I'm also using app
instead of container
for the main <div>
.
Anthony Albertorio
22,624 PointsAnthony Albertorio
22,624 PointsThis article should help on how to setup react on your local computer: https://www.codecademy.com/articles/react-setup-i