Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial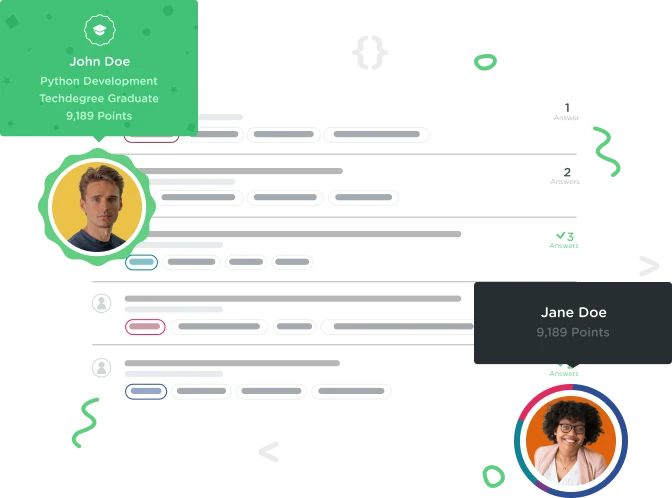
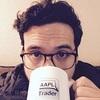
Nicholas Gaerlan
9,501 PointsWhere can I use arrow functions? and why are there extra parens?
here's a snapshot of my workspace. I've modified some of the code from the example and it works, BUT
I don't understand where I can use arrow functions in place of "function". I got the template literal thing down just fine and I like how I don't have to use so many concats and quotations marks. Another question I had is not directly related to this example but I've seen this sort of thing before
function ({
...some lines of code;
})();
what has me confused is that last set of parens. is that for error handling purposes? like if the function has no value to return, it will just alternatively behave like an empty function and the rest of the program will run anyways? That's my guess. If there's a video on this site that explains it, I'd love a link to it. I'm sure it's beyond the beginner level I'm at right now.
3 Answers
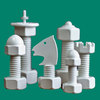
Steven Parker
231,198 PointsThe extra parentheses after the definition cause the function to be invoked. This syntax is known as an IIFE, or "Immediately Invoked Function Expression". I expect this is covered in one or more courses but I'm not sure exactly which ones.
Arrow functions can be used most places a function is needed, with a few restrictions. They cannot be used as constructors, and they don't automatically bind things like "this" so you must be sure the code in them won't depend on that. For a complete description of the differences, see this MDN page on Arrow Functions.
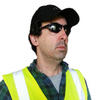
Phillip Kerman
Courses Plus Student 285 PointsThere's two pretty big topics here: IIFEs and arrow functions.
The IIFE is a little dated, but and while it can be hard to grasp there's not a whole lot there.
First, know that within a function ("...some lines of code") you can use all kinds of variables and functions and they're locked inside the function (that is, only apply to that scope). That's the first motivation for storing everything in there. You could have two IIFEs each with their own variables of the same name, yet they remain independent (which is good).
Notice your function is unnamed (that is, "anonymous"):
function(){
}
You could have just as easily named it myFunction:
function myFunction(){
}
In either case, you never arrive inside the function until you invoke it. If you wanted the second way, you could immediately invoke it manually, at the end:
function myFunction(){
}
//just invoke 'manually':
myFunction();
That will have the same effect as throwing the anonymous function inside parens, then throwing another paren at the end to invoke it:
(function(){
})()
That's basically the same as my "manual" invocation--but in my case, the myFunction would be in the global space (meaning you couldn't have another thing called myFunction). The important thing is that you forced all the code inside he function to execute and now it's part of your app. Without ever being invoked, it'd never "happen".
As for arrow functions, yes, they change the way function scope works and--perhaps more interesting but not as critical--is they make functions (including anonymous functions) nice and lightweight as far as syntax goes. In functional programming one big thing is the ability to pass functions around as parameters (that is, because functions are "first class" members of the language). Arrow functions make one-line functions easy and free from the need for the word "function" as well as the open and closing curly brace.
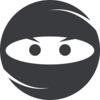
John Lundberg
20,368 PointsNicholas Gaerlan Rabbit holes makes life worth living :) Its the icing of the cake!
Nicholas Gaerlan
9,501 PointsNicholas Gaerlan
9,501 PointsGreat reading. I fell down a rabbit hole. Most of it beyond my current comprehension, but I understand a little of it. The main reason I see mentioned about IIFE is to keep variables from cluttering the global name space? Maybe that's an issue w/ "var" getting hoisted up into more global scope. Did some reading and learned that const and let solve some of the problem by limiting things to block scope, but if you are creating constructors or the module pattern (i'll have to watch this treehouse vid a few times) then "function" itself is not block scoped so you still have to do things the 'older' way. I guess things get better in ES2017 because I see that private fields are something in the pipeline https://vimeo.com/223283922 (starts at 17m:38ss). I come from a philosophy background so all the logic that goes into structuring the language is very interesting to me. JavaScript seems like a language designed to originally just make something happen (hence the "script"), but over time is trying to achieve more classically object oriented features. Meanwhile, modern languages all seem to be leaning into the functional programming space? All of that makes it sound like I might know what I'm talking about haha. Thanks for your answer and pointing me in the right direction.