Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial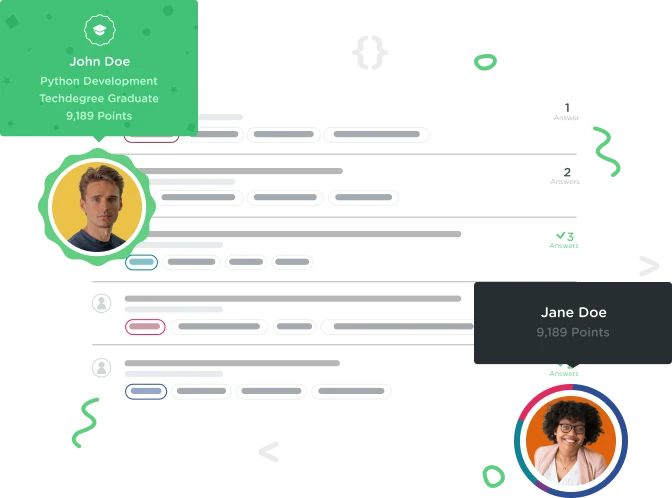

Todd Bascombe
Full Stack JavaScript Techdegree Student 11,133 Pointswhere did i go wrong ?
const express = require("express");
const router = express.Router();
const User = require("../models/User");
//get login/register
router.get("/login",(req, res, next)=>{
res.render("login");
});
router.post("/login", (req, res, next)=>{
res.json(req.body);
})
router.get("/register",(req, res, next)=>{
res.render("register", {
title: "Register"
});
});
router.post("/register",(req, res, next)=>{
if(req.body.fname &&
req.body.lname &&
req.body.email &&
req.body.password &&
req.body.password2){
if (req.body.password !== req.body.password2){
//Change for better functionality
var err = new Error("passwords do not matched")
err.status = 400;
return next(err);
}
const userData = {
usr_fname: req.body.fname,
usr_lname: req.body.lname,
usr_email: req.body.email,
usr_gender: req.body.gender,
usr_addr: req.body.addr,
usr_addr2:req.body.addr2,
usr_zip:req.body.zip,
usr_city:req.body.city,
usr_state:req.body.state,
password:req.body.password,
usr_major:req.body.major,
usr_DOB:req.body.DOB,
usr_phone:req.body.phone,
usr_OCC: req.body.Occ
//usr_gradYear: req.body.gradYear,
//usr_NonGrad: req.body.non_grad
};
//use the schema's creat method to add documtn into mongodb
User.create(userData, (error, user)=> {
if (error) {
return(error);
}else{
return res.redirect('/register/payment');
}
});
}else{
const err = new Error('All the * fields are required');
err.status = 400;
return next(err);
}
});
onst mongoose = require('mongoose');
const UserSchema = new mongoose.Schema({
usr_fname: {
type: String,
required: true,
trim: true
},
usr_lname: {
type: String,
required: true
},
usr_email: {
type: String,
unique: true,
required: true,
trim: true
},
usr_gender: {
type: String
},
usr_addr: {
type: String,
trim: true
},
usr_addr2: {
type: String,
trim: true
},
usr_zip: {
type: Number,
trim: true
},
usr_city: {
type: String,
trim: true
},
usr_state: {
type: String,
trim: true
},
password: {
type: String,
required:true
},
usr_gradYear: {
type: Date,
},
usr_major: {
type: String,
trim: true
},
usr_DOB: {
type: Date
},
usr_phone: {
type: Number,
trim: true
},
usr_OCC:{
type: String,
trim: true
}
});
const User = mongoose.model('User', UserSchema);
module.exports = User;
const express = require("express");
const bodyParser = require("body-parser");
const mongoose = require('mongoose');
const path = require('path');
const app = express();
//mongodb connection
mongoose.connect("mongodb://localhost:27017/ccnyaumni", {useNewUrlParser: true});
mongoose.set('useCreateIndex', true);
const db = mongoose.connection;
//mongo error;
db.on('error', console.error.bind(console, 'connection Error:'));
//body parser middleware
app.use(bodyParser.urlencoded({extended: false}));
//middleware for static files
app.use('/static', express.static("public"));
//pug install
app.set('view engine', 'pug');
//routes access
const mainRoutes = require('./routes');
const signupRoutes = require('./routes/signup');
//routes middleware
app.use(mainRoutes);
app.use(signupRoutes);
//error middleware
app.use((req, res, next)=>{
const err = new Error("Page Not Found");
err.status = 404;
next(err);
});
app.use((err, req, res, next)=>{
res.status(err.status || 500);
res.render('error',{message: err.message,
error:{}
});
});
const port = 3000;
app.listen(port,()=>{
console.log("server is starting");
});
MOD: Edited the question to format the code so it's more readable.
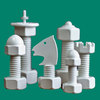
Steven Parker
229,744 PointsTo make it easier for other students to read your code, always use "markdown" formatting. There's a pop-up "cheatsheet" below, or you can watch this video on code formatting.
1 Answer
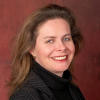
Niki Molnar
25,698 PointsA quick look at the only formatted code above, shows youβre missing a semi colon after the following line:
var err = new Error("passwords do not matched")
What error are you getting?
Niki
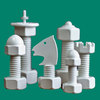
Steven Parker
229,744 Points Though it's "good practice" to use them, semicolons are optional at the end of a line unless "strict" mode is in use.
Todd Bascombe
Full Stack JavaScript Techdegree Student 11,133 PointsTodd Bascombe
Full Stack JavaScript Techdegree Student 11,133 Pointsi know it is alot