Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial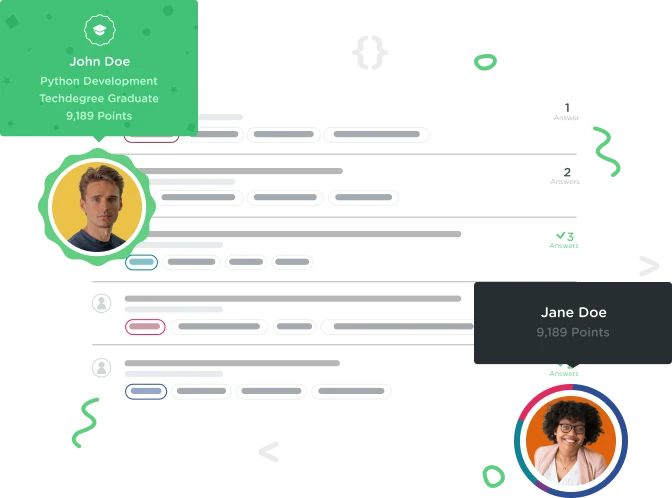
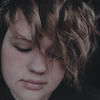
Ryan Maneo
4,342 PointsWhere did I mess up?
As you can see, I tried several ways of returning a String, and the instance just won't work.
enum MobilePhone /* I tried this */ -> String {
// I tried all 4 options I could think of too
case iPhone: return String
case Android return: String
case Blackberry: String
case WindowsPhone -> String
// Including this
return String
}
let iPhone = MobilePhone(iPhone: "6S")
2 Answers

Ryan Anderson
2,920 PointsWhen you're using an enum, you need to specify that you using a specific enum, and a specific case within the enum because the cases contain the information - the enum is kind of the container. The container can do some more advanced things, and it can specify what goes in the container, but it doesn't specifically have a value of its own.
So, let phone = MobilePhone doesn't really tell us anything, so you'll get an error. It's like saying direction = All the Directions instead of direction = north.
The stuff that comes after the dot is the "argument." So you're saying let this thing = the enumeration "MobilePhone" and within that, the case "iPhone." Kind of like a dropdown menu where you choose MobilePhone, and then select iPhone from the list. You can't just select MobilePhone, because it's just a container.
There is a chance at some point you'd want to refer to the type case with MobilePhone.self, but I wouldn't worry about that for now.
Hope that helps.

Ryan Anderson
2,920 PointsYou're close - the syntax for an associated value is:
case Thing(Type)
In this case, you want to declare an associated value of type String for the iPhone case.
enum MobilePhone {
case iPhone(String)
case Android
case Blackberry
case WindowsPhone
}
Then, to assign it to a constant, you need to add the string after the instance of MobilePhone with case iPhone:
let iPhone = MobilePhone.iPhone("6S")
Hope that helps.

Martin Wildfeuer
Courses Plus Student 11,071 PointsJust to follow up on this: the syntax for creating an enum that returns a String (or any other type) would be:
enum MobilePhone: String {
case iPhone = "iPhone"
case Android = "Android"
...
}
let iPhone = MobilePhone.iPhone.rawValue // "iPhone"
Not related to the challenge and not a solution ;)
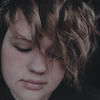
Ryan Maneo
4,342 PointsWhy dot notation? I thought you would call an instance of it... really confused now in general. Thanks for explaining though.
Ryan Maneo
4,342 PointsRyan Maneo
4,342 PointsGreat explanation... definitely helped. Thanks!