Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial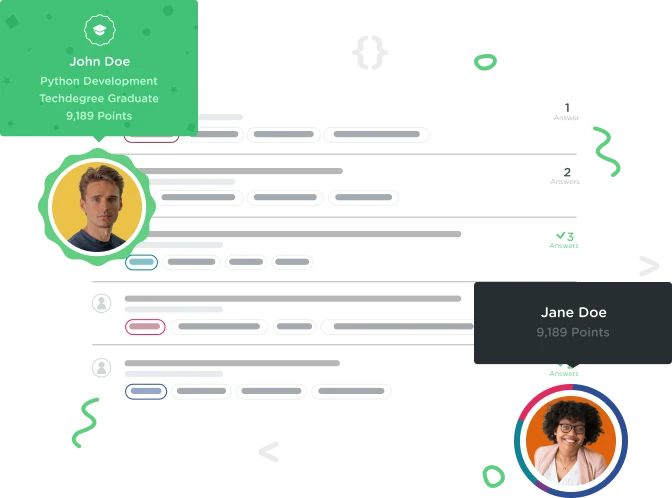
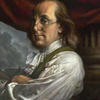
Jeff Busch
19,287 PointsWhere did this variable come from?
Hi Treehouse,
I'm a little confused. I going through a php book, The Joy of php Programming, and it's building a used car website using a mySQL database. In one of the files (ViewCars.php) there is a query. In the file is a while loop to print the results to the screen. There is a variable $result_ar being used and I don't understand where it comes from (where it is being declared). ViewCars.php also calls (include) a file named db.php. Below is the code. If someone can clear this up for me I'd really appreciate it.
Thanks, Jeff
/*********** ViewCars.php **********************/
<body>
<div id="main-wrapper">
<h1>Sam's Used Cars</h1>
<h3>Complete Inventory</h3>
<?php
include 'inc/db.php';
$query = "SELECT * FROM INVENTORY";
/* Try to insert the new car into the database */
if ($result = $mysqli->query($query)) {
// Don't do anything if successful.
}
else
{
echo "Error getting cars from the database: " . mysql_error()."<br>";
}
//***
echo "<table id='Grid'><tr>";
echo "<th style='width: 50px'>Make</th>";
echo "<th style='width: 50px'>Model</th>";
echo "<th style='width: 50px'>Asking Price</th>";
echo "</tr>\n";
$class ="odd";
while ($result_ar = mysqli_fetch_assoc($result)) {
echo "<tr class=\"$class\">";
echo "<td class=\"$class\" style='width: 50px'>" . $result_ar['Make'] . "</td>";
echo "<td class=\"$class\" style='width: 50px'>" . $result_ar['Model'] . "</td>";
echo "<td class=\"$class\" style='width: 50px'>" . $result_ar['ASKING_PRICE'] . "</td>";
echo "</tr>\n";
if ($class=="odd"){
$class="even";
}
else
{
$class="odd";
}
}
echo "</table>";
$mysqli->close();
include 'inc/footer.php'
?>
</div>
</body>
/**************** db.php ************************/
<?php
$mysqli = new mysqli('localhost', 'jeff', 'random', 'Cars' );
/* check connection */
if (mysqli_connect_errno()) {
printf("Connect failed: %s\n", mysqli_connect_error());
exit();
}
?>
8 Answers
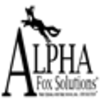
Shawn Gregory
Courses Plus Student 40,672 PointsHello,
Guys just going to clear some things up. As far as the $result_ar goes, Dean is right, the variable gets created when the parser comes to the while loop. Once the code $result_ar = mysqli_fetch_assoc($result) gets ran, what's returned gets put into the variable which then becomes an array as the information returned from the function mysqli_fetch_assoc() is an array. Then you use the new array inside of the while loop or anywhere else in your code afterwards.
The 'condition' statement for the while loop will be true so long as the function mysqli_fetch_assoc() returns something. Once mysqli_fetch_assoc() cannot return anything, it returns false. Basically the line:
while($result_ar = mysqli_fetch_assoc($result))
is the same as:
$result_ar = mysqli_fetch_assoc($result);
while($result_ar) { // Or while(isset($result_ar))
/* Code goes here... */
}
Basically the reason why it's inside of the while loop is because since there maybe multiple rows in the database to return, you'll have to call the function over and over again and it will continue to return one row a time depending on the query that was sent to the database. You are not only looping through the results, but also looping through the database information to return the information to use.
The while loop may or may not get used if nothing gets returned from the database. If there is something in the database and it gets returned, it's considered true and the while loop gets used. Additionally, if you want a while loop to be used at least once, you would use a do...while loop. I hope I was able to help you (even if it's about 7 hours late :D ).
Cheers!
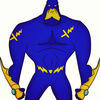
I'm Dean Carter
3,383 PointsThe variable is declared at the beginning of the while loop, if I'm not mistaken.See theline of script below.
while ($result_ar = mysqli_fetch_assoc($result)) { ...
From my limited PHP exprience... The loop is saying that while the variable $result exists (once a car - and it's info - is being created to the database and $result is not null), then it will then be known as the variable $result_ar and output/ apply the make, model and asking price info.
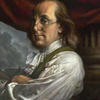
Jeff Busch
19,287 PointsWhat's confusing me is that a while loop will run as long as the condition in the parenthesis is true. How is the condition considered true if $result_ar has no value assigned to it before the loop begins? while, php.net
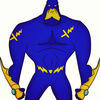
I'm Dean Carter
3,383 PointsAs I tried to explain previously, from what I can tell, the values from $result (if any are in the database) are passed to $result_ar in the same instance it is declared at the beginning condition of the loop.
I assume $result would be null until a successful query was made at which point the code would create $result_ar and pass it's values temporarily from $result, then run the loop and it's corresponding variables called from the database to 've displayed and once the script passed through the while loop then $result_ar values would return to null thus halting the loop and outputting the data for the car.
All this is started and completed at the conditional argument line at the beginning of.the while loop.
Hopefully that is clearer. As I said I may be wrong, but that is what I see in the script
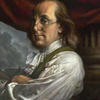
Jeff Busch
19,287 PointsHi Dean,
I appreciate the time you've given me. I'm still having trouble wrapping me head around this because the while loop checks the condition before the block is executed. Also, sometimes if the while expression evaluates to FALSE from the very beginning, the nested statement(s) won't even be run once. I just don't understand how the condition is true to begin with. Maybe I just need to sit back and let it sink in.
Thanks Again,
Jeff
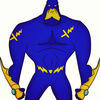
I'm Dean Carter
3,383 PointsThank you That was a way clearer and eloquent way of saying what I see when I look at the code.
I haven't had my PHP Erika moment yet. Lol.
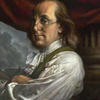
Jeff Busch
19,287 PointsI think one of my problems was looking at ($result_ar = mysqli_fetch_assoc($result) as a comparison when it is an assignment. $result_ar isn't being compared to mysqli_fetch_assoc($result), it's receiving a value, same in the if statement. $result_ar is assigned value from mysqli_fetch_assoc($result) and $result was assigned values (the indexes of $query) in the previous if statement, which had gotten a value from $mysqli->query($query), and $query was assigned a value from $query = "SELECT * FROM INVENTORY";.
Am I on the right track?
Thanks Guys,
Jeff
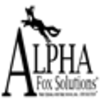
Shawn Gregory
Courses Plus Student 40,672 PointsJeff,
Take a look at the following code. This is the sequence of lines I use when I communicate with my MySQL database to see if a user is in the database by their username and password. Please note that I use MySQLi a little bit differently.
$db = new mysqli(xxxxxxxxxx, xxxxxxxxxx, xxxxxxxxxx, xxxxxxxxxx);
$result = $db->query("SELECT * FROM users WHERE user='$user' AND pass='$pass'");
while($row = $result->fetch_assoc()){
if($result->num_rows > 0) {
/* There's a user in the database that matches the username and password provided. */
}
}
Line 1 opens a connection to the MySQL database. Note that because we used the 'new' keyword, we are dealing with objects.
Line 2 sends the query to the database and returns a pointer to the query that you can use to either extract the data or find out information about the query like the amount of rows that match the query, etc.
Line 3 is the while loop that contains the function that returns the data from the database and puts it into the $row array.
Line 4 uses the $result pointer and uses the property num_rows that returns the amount of rows that can be returned that matched the query and makes sure that at least one row will be returned.
So to put it in summary: $db is the connection to the database, $return is the pointer of the query returned where you can extract or obtain information about the query, $row is the array that will contain the data from the database, and num_rows is the property that returns the number of rows that match the query.
Of course I would never use the while loop when checking if a user is in the database, I am just using it as an example to the example the OP provided. I hope this gives you a little bit more information that you are looking for.
Cheers!