Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial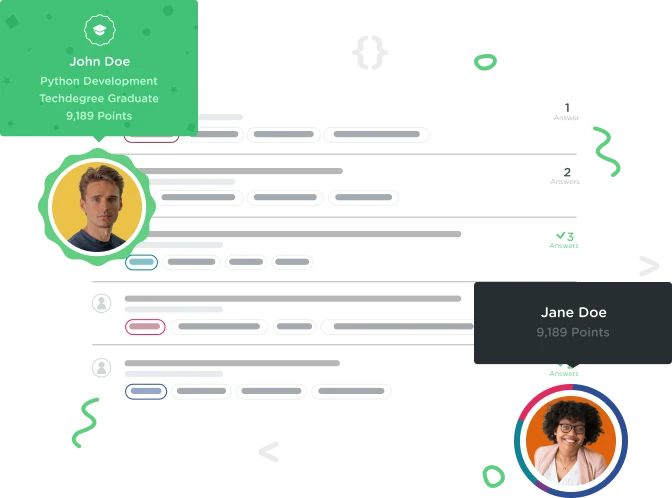

Abe Daniels
Courses Plus Student 2,781 PointsWhere do i put the "m"s!
I feel like i have constructed a proper constructor. I am getting a bummer on this because it is unhappy with my placement of "m." Can anyone help? Thanks! :D
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (!mFieldName.indexOf(0) = 'm' && mFieldName.isUpperCase(1) ) {
throw new IllegalArgumentException(mFieldName + "is not valid fieldName");
}
return fieldName;
}
}
3 Answers
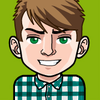
Harry James
14,780 PointsHey Abe!
You're trying to check the values of mFieldName
rather than fieldName
and, that will give you a few errors.
Also, you're checking to see if at index 0 the character is not m
and also if at index 1 it is uppercase - it needs to fail either way, not just if both cases are met and, you want to display the exception if the character at index 1 is lowercase instead.
As well as this, I'd recommend you use the method .charAt()
when dealing with characters as you may get some errors otherwise.
Hope it helps and if you have any more problems, give me a shout :)
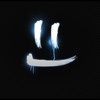
Grigorij Schleifer
10,365 PointsYou can use this code for validation of the first char:
I created a char variable "mChar" and stored the 'm' char inside of it, so i could use the != statement
public static String validatedFieldName(String fieldName) {
char mChar = 'm';
if(fieldName.charAt(0) != mChar){
throw new IllegalArgumentException("he second letter in the field name must be uppercased");
}
For the validation of the second character you can use this
if(! Character.isUpperCase(fieldName.charAt(1)) {
}
How to use the isUpperCase() method google here:
stackoverflow.com/questions/4452939/find-if-first-character-in-a-string-is-upper-case-java

Abe Daniels
Courses Plus Student 2,781 Pointspublic class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
char mChar = 'm';
if(fieldName.charAt(0) != mChar) {
throw new IllegalArgumentException("Your must start your field with an 'm.'");
}
if(Character.isLowerCase(fieldName.charAt(1))) {
return fieldName;
}
}
}
it says i am missing a closing curly brace. I put one in, and it tells me "class, interface, or enum expected." What do i do?
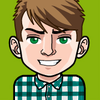
Harry James
14,780 PointsHey again Abe!
At the end of the method validatedFieldName()
(Above the 2nd last curly brace), you want to return fieldName
.
Also, if the character at index 1 is lowercase, you want to throw an exception, rather than returning the fieldName.
Hope it helps and if you have any more problems, give me a shout :)
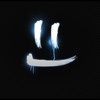
Grigorij Schleifer
10,365 PointsYou are nearly done ... your validatedFieldName method must return fieldName. As Harry said :)
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
char mChar = 'm';
if (fieldName.charAt(0) != mChar) {
throw new IllegalArgumentException("Your must start your field with an 'm.'");
}
if(Character.isLowerCase(fieldName.charAt(1))) {
throw new IllegalArgumentException("The secon letter must be uppercase !");
}
return fieldName;
}
}
Keep on rockin !!!!
:)