Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial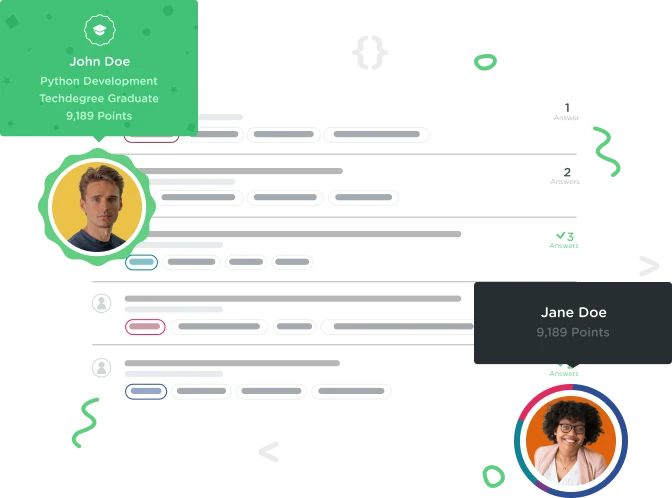
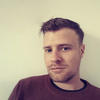
Jaco Kotzee
Courses Plus Student 20,157 PointsWhere does taskListItem come from and what does it do?
I am a bit confused as to what the purpose of taskListItem does int the below context.
I am also unsure of what the '("input[type=checkbox]");' does, and the button.edit
var bindTaskEvents = function(taskListItem,checkBoxEventHandler);{
console.log("Bind list item events");
// select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.edit");
// bind editTask to edit Button
// bind deleteTask to delete button
// bind checkBoxEventHandler to checkbox
}
Would appreciate some insights into this.
2 Answers
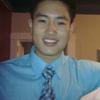
Rui Bi
29,853 PointsIn this case, taskListItem is just a function parameter. A JavaScript parameter is just a placeholder for storing arguments passed to the function when the function is called.
Perhaps its confusing because for some reason, he is using JavaScripts alternative function declaration method which is:
var functionName = function(params...){
}
Which is exactly the same as:
function functionName(params...){ }
If it looks more familiar, then the above could could actually be written:
function bindTasEvents(taskListItem, checkBoxEventHandler){ }
The taskListItem parameter is a placeholder for a collection of DOM objects that would be passed to the function as an argument
The next two items, ("input[type=checkbox]") and ("button.edit") are just CSS selectors, wrapped in quotes to make them valid JavaScript strings.
input[type=checkbox] selects all input tags with the type attribute equal to checkbox. In order words, it selects all checkbox form elements.
button.edit selects all button tags with the class of, "edit." So it selects all the button elements that had the class, "edit," applied in the HTML markup.
These selectors are used with the .querySelector() function, which takes as an argument a string representing a css selector and returns the first DOM object/element that matches the given selector.
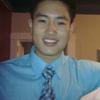
Rui Bi
29,853 PointsIn that context, taskListItem is still the parameter that was passed to the function. However, based on how it is used, it would have to be a DOM element. They querySelector method is then called on that DOM element in order to return the first DOM element that is a checkbox. The result is cached into the variable checkbox.

Gabriel Ward
20,222 PointsOk, but how do we know taskListItem a DOM element? Because it seems like it's an arbitrary name. It's not like the more familiar elements such paragraph, anchors, and headers right? I guess I'm just struggling to get my head around it because it seems so arbitrary and random.
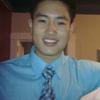
Rui Bi
29,853 PointsThe name is arbitrary. listTaskElement is being passed into the bindTaskEvents function as a parameter. Within the function, listTaskElement refers to whichever variable was passed into the function. It's kind of like a placeholder. The name could have been anything: if you changed all references of listTaskElement to "myElement", for example, the function would work the same. The reason you know that listTaskElement is a DOM element is because the querySelector method can only be called by DOM elements. If, for instance, you passed in a non-DOM element as listTaskElement to the bindTaskEvents function, then your code would throw an error because only DOM elements can use the querySelector method.
When you actually call (use) the bindTaskEvents function, you would replace listTaskElement with a real DOM element you want to pass to your function as an argument. Your call could look like this:
var myTaskItem = document.querySelector('form'); var myCheckBoxEventHandler = function(){...}; var bindTaskEvents = function(myTaskItem, myCheckBoxEventHandler);

Gabriel Ward
20,222 PointsOk, so I think I've got it: in this situation the bindTaskFunction isn't actually being called, it's just being defined. And so at this stage taskListItem can be an arbitrary thing that isn't actually an element. Is this a correct interpretation?
Thank you so much for your help.
Jaco Kotzee
Courses Plus Student 20,157 PointsJaco Kotzee
Courses Plus Student 20,157 PointsGreat! That Helps me a lot!
Gabriel Ward
20,222 PointsGabriel Ward
20,222 PointsHi Jacob and Rui,
I've been watching this video and was confused about where taskListItem fits in in all this. I get that it is a placeholder parameter in the bindTaskEvents function, but I'm unsure how it is then used as an element in the querySelector syntax. Would you be able to explain how it goes from being a parameter to an actual element? My understanding is that in the following code,
var checkBox = taskListItem.querySelector('input[type=checkBox]');
taskListItem is an element.
Thanks in advance for your help.