Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial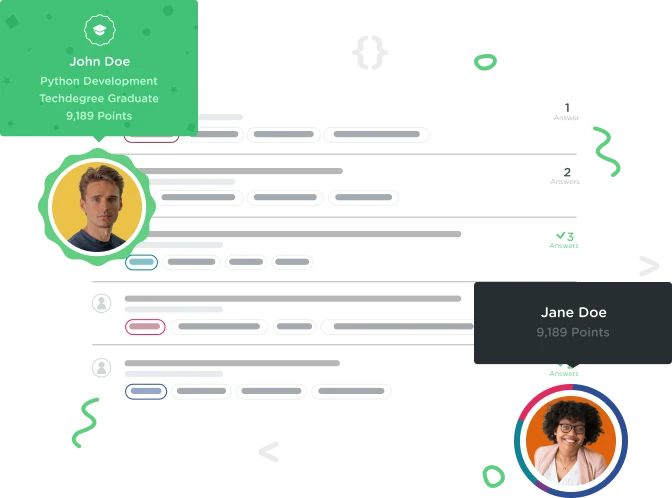

Robert Muldoon
4,892 PointsWhere is the connection between the Song and Playlist objects?
A few people expressed confusion around the play() and stop() functions being called on the song within the array of playlist songs. I have a similar question, but it hasn't been answered by the existing comments
In the below code, we define the Playlist constructor function, and one of the properties is an array of songs. I understand that this array is meant to be a collection of Song instances, but where exactly is that relationship defined?
function Playlist() {
this.songs = [];
this.nowPLayingIndex = 0;
}
Then, when we use the below code to define the variable "currentSong" how does the program know that the variable has anything to do with the Song constructor? As far as I can tell, all we're doing is assigning it to an array within the Playlist constructor, but I don't see a connection back to the Song constructor
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPLayingIndex];
currentSong.play();
};
Finally, when we call the commands "currentSong.play()" and "currentSong.stop()" I am confused as to how the program understands that currentSong is allowed to utilize the play() and stop() functions that are defined in the Song constructor, if there's no relationship established anyway in the code
Thanks very much for clearing this up
1 Answer
Seth Shober
30,240 PointsAdmittedly I haven't taken this course, so I might be missing some pieces, but I think I understand the overall logic as to what is being accomplished. Prototype chains can definitely be confusing at times, but are important to master because they are the power and beauty of JS. Here we have a Playlist constructor function that you have defined at the top.
When you create a Playlist with the new
keyword like, myPlaylist = new Playlist()
, it adds the properties of the Playlist constructor to the myPlaylist
object and gives access through prototypical inheritance to the prototypes of Playlist. What the new
keyword also does is create a new this
value, which in our case will associate the context of this
with myPlaylist
. You mention the songs array inside of Playlist and being curious about how it is associated with the Song constructor. Well, it actually isn't associated at all, but rather each song inside the array is.
You see, each song in the array was created by new Song()
, and that means each song has access to the play
and stop
prototypes given by the Song constructor. What the currentSong
variable is actually doing is grabbing a specific Song object (or child of Song).
So, in the end, we are looking up the Song prototype chain, not the Playlist prototype chain, and calling play()
on Song. Again forgive me if I'm missing something from the exercises, or if I'm not completely grasping this, but does this make sense?

Robert Muldoon
4,892 PointsSeth, it took me some time to follow your logic, but I finally understand this! Your explanation was exactly what I needed, so thank you for taking the time to help me with this
For anyone else still following this logic, below is the code in which we use the "new Song()" construct that Seth mentioned in his original answer. It now makes sense to me why each song within the songs array has the properties and methods in the Song constructor
var playlist = new Playlist();
var hereComesTheSun = new Song("Here comes the Sun", "The Beatles", "2:54");
var walkingOnSunshine = new Song("Walking on Sunshine", "Katrina and the Waves", "3:43");
playlist.add(hereComesTheSun);
playlist.add(walkingOnSunshine);
Seth Shober
30,240 PointsGlad I could clear that up for you. Thanks for providing some visuals for my thoughts.

Sanjay Tailor
8,443 PointsThank you Seth for this explanation... This clarified my confusion... Thanks!
Sanjay Tailor
8,443 PointsSanjay Tailor
8,443 PointsThank you for asking this. I had the same confusion... Great answer as well. Thank you