Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial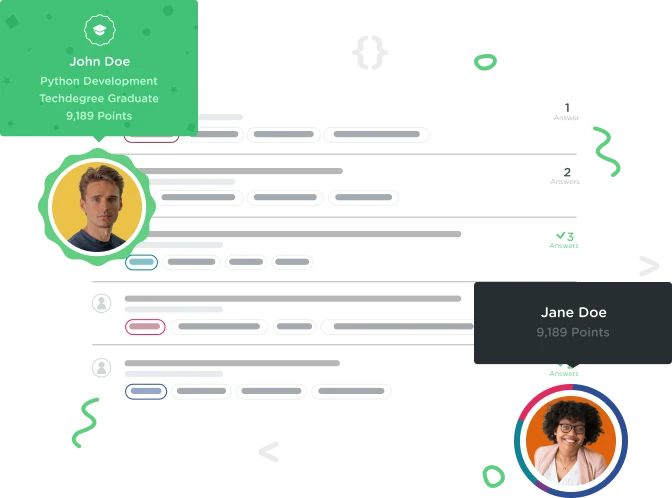
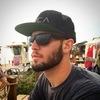
Morgan Walstrom
4,683 PointsWhere is the function being invoked?
var upper = 10000; var randomNumber = getRandomNumber(upper); var guess; var attempts = 0;
function getRandomNumber(upper) { return Math.floor( Math.random() * upper ) + 1; }
while( guess !== randomNumber ){ guess = getRandomNumber( upper ); attempts += 1; } document.write("<p>The random number was: " + randomNumber + "</p>"); document.write("<p>It took the computer " + attempts + " attempts to get it right.</p>");
2 Answers

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsMorgan Walstrom , you can see in 2nd line of your code i,e :
var upper = 10000;
var randomNumber = getRandomNumber(upper); // Function getting invoked here
var guess;
var attempts = 0;
function getRandomNumber(upper) {
return Math.floor(Math.random() * upper) + 1;
}
while (guess !== randomNumber) {
guess = getRandomNumber(upper);
attempts += 1;
}
document.write("<p>The random number was: " + randomNumber + "</p>");
document.write("<p>It took the computer " + attempts + " attempts to get it right.</p>");
So , you can see in 2nd line , function is getting invoked and the retured value gets stored into the variable randomNumber.
Actually this is called Hoisting .
In JavaScript , both functions declaration and function definition get hoisted.
In other words , JavaScript interpreter allows yo to use the function before the point at which it was declared in the source code.
Hope it help :)

Ivy Garrenton
3,772 PointsSo we can call a function before the function is written in the script?
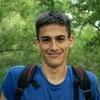
Anastasios Poursaitedes
10,491 PointsWe can call a function before it is declared yes, that's hoisting as Aakash said above, although we can call a function we can't call a function expression. You should avoid hoisting as much as possible. Things could get really complicated really fast.
Morgan Walstrom
4,683 PointsMorgan Walstrom
4,683 PointsI thought so, I just have never invoked a function that was being stored in a variable. var upper = 10000; var randomNumber = getRandomNumber(upper);
so on line 2 is the parameter the variable on line one? upper is getting passed in? cool way to do it just new to me!
Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsAakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsActually , the function is not stored in a variable . What's going on is -
We are calling the function first , then storing it's value in a variable . So ,it's the value that gets stored not the function itself. Remember ,whenever a function returns a value , we want to store that value in some variable so that we can use that value.
Same thing is going on here , function is returning a value and we are so storing that value in a variable in line 2.
Morgan Walstrom
4,683 PointsMorgan Walstrom
4,683 PointsThanks that makes a lot of sense!