Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial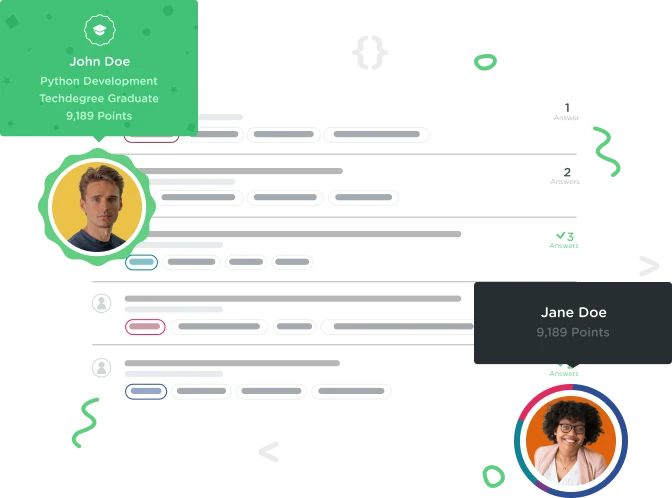
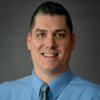
Darrin Fagley
12,432 PointsWhere is the lower number?
Okay so I thought about this equation a little differently in my head. I am not fully understanding the explanations in some of the other questions about how the range is determined, but the math made sense to me if I thought about it like this:
For example, if we use 1 as the lower and 10 as the upper: Math.floor(Math.random() * (upper - lower) + 1) + lower;
So if we have 10 - 1 that equals 9. Math.random() will go up to but not include 9 so we add plus 1 giving us 10 in which case Math.random() gives us up to but not including 10. Then we add lower, which then allows the upper number to be included inclusively. I understood and got all that. However, while experimenting with the full code and different value sets, I ran across something I did not understand.
const getRandomNumber = (lower, upper) => { return Math.floor(Math.random() * (upper - lower) + 1) + lower; };
// Call the function and pass it different values console.log (getRandomNumber(1, 10)); console.log(getRandomNumber(1, 3));
Using the code above, which as far as I can tell is the same as in the video's except it is an arrow function, I ran the console.log(getRandomNumber(1, 10)); probably 50 times and got every number but the lowest number of 1, it never came up even once. This was further proven when I ran console.log(getRandomNumber(1,2); which should be either a 1 or a 2 and every single time I run it I only get 2. So I am confused as to how we are determining the lower number and why it is not being included in my tests?
1 Answer
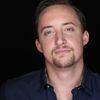
Blaize Pennington
13,879 Pointsconst getRandomNumber = (lower, upper) => { return Math.floor(Math.random() * (upper - lower) + 1) + lower; };
The problem you are having is with order of operations. Let's look at your final code without variables.
Math.floor(Math.random() * (50 - 1) + 1) + 1;
//First thing is the parentheses, so 50 - 1 = 49;
Math.floor(Math.random() * 49 + 1) + 1;
//This is where the error is, because of order of operations, you Multiply and Divide before you Add and Subtract.
//So the next thing you do is calculate the Math.random(); And let's say you hit the lowest number possible.
Math.floor(0 + 1) + 1;
//This as you can see results in 2 being your answer, not 1
If you add the +1 inside the parentheses, it changes everything:
Math.floor(Math.random() * (50 - 1 + 1)) + 1;
//First thing is the parentheses, so 50 - 1 + 1 = 50;
Math.floor(Math.random() * 50) + 1;
//The lowest possible result
Math.floor(0) + 1;
0 + 1 = 1;
//The highest possible result
Math.floor(49.99999) + 1;
49 + 1 = 50
Darrin Fagley
12,432 PointsDarrin Fagley
12,432 PointsBlaize Pennington, Ah!!!!! I see it now. Thank you for clearing that up, it was definitely causing some confusion on my end.