Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial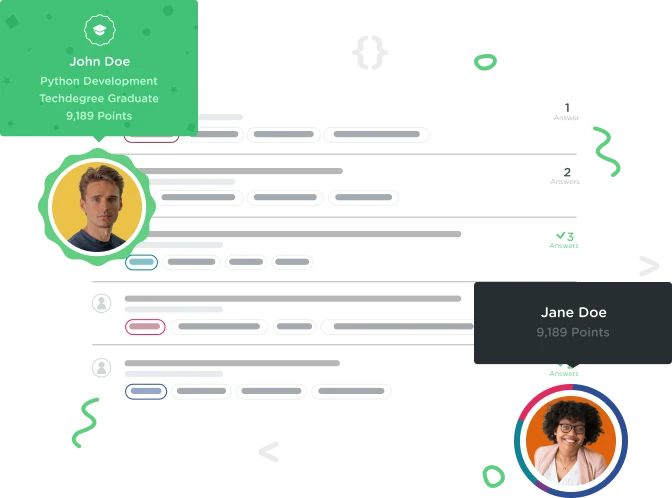

Tin Trucek
2,628 Pointswhere is the mestake
i cant see the mastake
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature > 21)
{
Console.WriteLine("Too Cold!");
}
if else(temperature == 21 || temperature == 22)
{
Console.WriteLine("Just right.");
}
else
{
Console.WriteLine("Too hot");
}
6 Answers

Axel McCode
13,869 PointsTry removing the semi-colon after the else if condition
else if (temperature == 21 || temperature == 22) // <-- no semi-colon

Clinton Hopgood
7,825 Pointselse if {}
not
if else {}
https://msdn.microsoft.com/en-us/library/5011f09h.aspx#Anchor_1
Your first > needs to be < and add an ! to the end of too hot

Tin Trucek
2,628 PointsThis is the error i get:
StudentsCode.cs(14,1): warning CS0642: Possible mistaken empty statement
StudentsCode.cs(17,0): error CS1525: Unexpected symbol `else'
Compilation failed: 1 error(s), 1 warnings
The code i wrote: string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature < 21)
{ Console.WriteLine("Too Cold!"); }
else if (temperature == 21 || temperature == 22);
{ Console.WriteLine("Just right."); }
else
{ Console.WriteLine("Too hot!"); }

Jannung Kusdiantoro
6,743 Pointstry my code
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
Console.WriteLine("Too Cold!");
}
if else(temperature >= 21 || temperature <= 22)
{
Console.WriteLine("Just right.");
}
else
{
Console.WriteLine("Too hot");
}
it is better to use double as input, because the range from 21 to 22 was less than one, so use double.

Tin Trucek
2,628 PointsStudentsCode.cs(14,3): error CS1525: Unexpected symbol else', expecting
('
StudentsCode.cs(15,0): error CS1525: Unexpected symbol {'
StudentsCode.cs(18,0): error CS1525: Unexpected symbol
else'
Compilation failed: 3 error(s), 0 warnings
that is what i get

dsteelz
1,203 PointsHello again, sorry about my previous response, i had no idea how to properly send code as a message. THIS is the proper code you can look at
class Program
{
static void Main(string[] args)
{
/*you should always prompt the user beforehand if you want information
when i ran your code, i thought nothing was happening because I was not prompted to enter a value*/
Console.WriteLine("Hello, what's the weather like today? enter a temperature value to find out.");
string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature < 21)
{
Console.WriteLine("Too Cold!");
}
else if (temperature >= 21 && temperature <= 22)
/* you wrote "if else"....this is invaild. the proper way is "else if"
* also, you were using the wrong operator. || means "or". what you had was the equivalent of
* temperature >= 21 OR temperature <=22. you want &&, which means "and" this tells the complier
* that temperature must be between 21 AND 22*/
{
Console.WriteLine("Just right.");
}
else
{
Console.WriteLine("Too hot");
}
}
}

Tin Trucek
2,628 PointsStudentsCode.cs(7,4): error CS1525: Unexpected symbol class'
StudentsCode.cs(8,4): error CS1525: Unexpected symbol
{'
StudentsCode.cs(9,8): error CS1525: Unexpected symbol static'
StudentsCode.cs(9,16): error CS1547: Keyword
void' cannot be used in this context
StudentsCode.cs(9,24): error CS1525: Unexpected symbol ('
StudentsCode.cs(38,0): error CS1525: Unexpected symbol
}'
Compilation failed: 6 error(s), 0 warnings
this is what i get when i run your cod.
And i think i wont the message to write out if the temperature is 21 or 22, because if there is an AND operation it means it has to be 21 and 22 in the same time.

dsteelz
1,203 Pointsthe code was written in Visual Studio, the IDE i use for c#. there may be some things that work in Visual Studio and not in Mono. also, the && operator won't make it so that temperature has to be 21 and 22 at the same time; rather, it will make it so that temperature can only be between 21 and 22.

dsteelz
1,203 PointsI rewrote your code. please read all of my comments within the code. it will save you some headaches in the future. make the necessary adjustments to your code.
namespace TreeHouseTest { class Program { static void Main(string[] args) { /you should always prompt the user beforehand if you want information when i ran your code, i thought nothing was happening because I was not prompted to enter a value/ Console.WriteLine("Hello, what's the weather like today? enter a temperature value to find out.");
string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature < 21)
{
Console.WriteLine("Too Cold!");
}
else if (temperature >= 21 && temperature <= 22)
/* you wrote "if else"....this is invaild. the proper way is "else if"
* also, you were using the wrong operator. || means "or". what you had was the equivalent of
* temperature >= 21 OR temperature <=22. you want &&, which means "and" this tells the complier
* that temperature must be between 21 AND 22*/
{
Console.WriteLine("Just right.");
}
else
{
Console.WriteLine("Too hot");
}
}
}
}

Tin Trucek
2,628 PointsNow i understand, if i use the AND operation the number will be in the range from 21 to 22, but if i use OR the number could be 50. thanx
Tin Trucek
2,628 PointsTin Trucek
2,628 Pointsit workt good now, thanks