Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial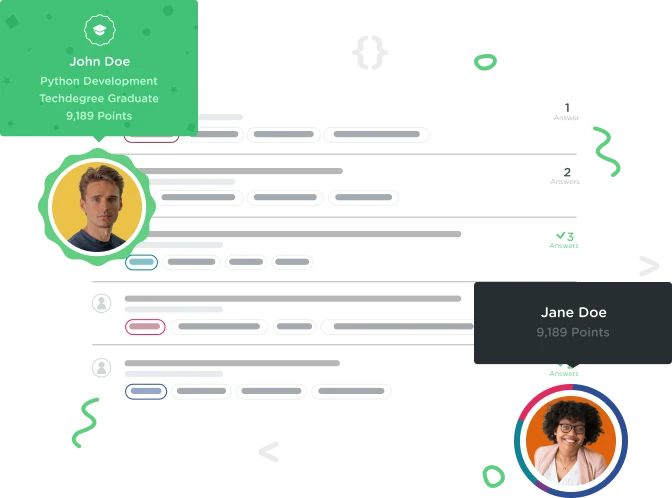

Jan Vrzal
3,687 PointsWhere is the problem ?
error: The setter method for fahrenheit
did not assign the right value to the celsius
property. (Note: Celsius = (Fahrenheit-32)/1.8)
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get{
return (celsius * 1.8) + 32.0
}
set{
celsius = (fahrenheit-32)/1.8
}
}
}
2 Answers
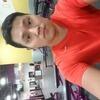
Tommy Choe
38,156 PointsHey Jan,
don't forget to use the special keyword "newValue" to get the value that is being set. Replace "fahrenheit" with "newValue" and you should be able to pass the challenge.
Hope that works!
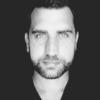
Jhoan Arango
14,575 PointsHello there
- Computed Properties
βIn addition to stored properties, classes, structures, and enumerations can define computed properties, which do not actually store a value. Instead, they provide a getter and an optional setter to retrieve and set other properties and values indirectly.β
So we know that a computed property is just a read-only property, where you canβt capture the value and use it somewhere else, it ONLY computes something and displays it (if you can say it like that).
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get {
return (celsius * 1.8) + 32.0
}
set {
celsius = (newValue - 32)/1.8
}
}
}
var temp = Temperature()
temp.fahrenheit = 89 // newValue being passed
In this challenge in order for us to set a value to the celsius property we can use the newValue keyword, this will set the value passed through the fahrenheit computed property.
Hope you understand a bit more.