Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial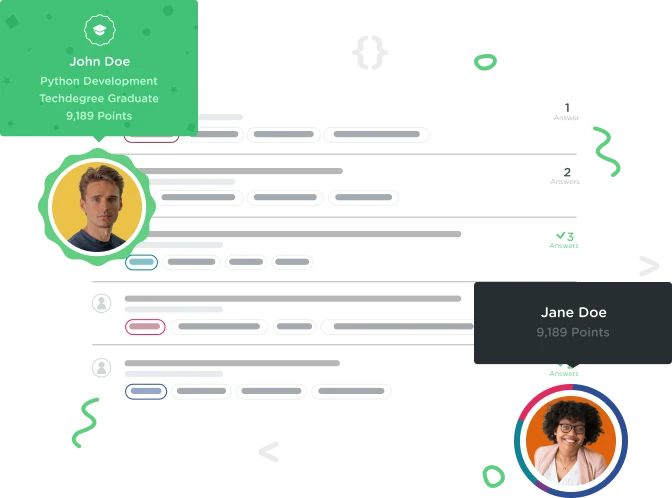

nicolasverger3
3,451 PointsWhere is the return keyword ?
Is the return keyword optional in Ruby ?
I thought that when a method returned a variable, you had to write "return" before the variable name.
But it seems that writing "return" is optional in Ruby.
Can anyone here confirm that ? Jason failed to explain this point in the video.
2 Answers
William Li
Courses Plus Student 26,868 PointsThere's another case when you must use return
keyword in Ruby.
def return_args(a, b)
a , b # wrong
end
def return_args(a, b)
return a , b # correct
end
When a function has multiple return values, the use of return
keyword is required here.
I have a very different view on this discussion. I suggest that after learning how return
keyword and implicit return work in Ruby, you should get into the habit of omitting return
when not required while writing Ruby code. The reason is simple, because this is the coding style the entire Ruby community has agreed upon. You may check out Github's Ruby styling guideline for more reading.
Every programming language has its own styleguide, it often contains a lot of best practices & coding style on how to write better code in that particular language. It might be tempting to bring your old habit from another language over and write the code in whatever style you think it's best, that's a bad idea, it's like moving to a new country but not making an effort to adapt to that country's culture or tradition.
Let me tell you a little story. Some years ago, I was collaborating on a Ruby project with a small team. I made a pull request to refactor a Class by extracting some duplicated code into a helper method. Sth like this.
def helper v1, v2
# method body
end
This pull request was turned down during the code review because while the change I made was good, the other members of the team didn't like the style there, they insisted that I write it this way instead.
def helper(v1, v2)
# method body
end
In today's world, programming project is seldom a solo effort, you're writing the code for yourself as much as writing it for others to read. Therefore, all contributors write the code by following one set of coding style rule helps to make the entire codebase cohesive, readable, and maintainable.
Kind of. Ruby has a somewhat unique feature "implicit return"
using return is a good habit to get into if you ever intend to program in other languages since most will require you to explicitly use the return keyword.
I should point out that implicit return isn't unique to Ruby. While many languages (C, Java, Python) only allow explicit return by writing a return
statement; in the world of functional programming languages (lisp, Clojure, Scheme ... etc), the return
keyword is gone, every function definition uses implicit return. In particular Lisp, a programming language that's almost 60 years old (way older than C), is highly influential in the creation of Ruby, Ruby's creator Matz borrowed many FP concepts from Lisp and implemented 'em into the language, implicit return is one of them.
Hope this helps clear things up.

andren
28,558 PointsKind of. Ruby has a somewhat unique feature "implicit return" where a function will automatically return the last value it handled even if you don't use the return keyword. So if you have some value you want to return at the end of a function then you technically don't have to use return. For example:
def addTwoNumbers(a, b)
a + b
end
That is a valid function. It will return the result of a + b
even though the return keyword is not used, since that is the result of the last operation that takes place in the function.
However return
still has its uses, if you want to return a value in the middle of a function for instance, as part of some loop or conditional statement then you have to use return
. I personally also think that using return
even when it isn't mandatory is still good practice because it is arguably easier to read what the code does. And using return
is a good habit to get into if you ever intend to program in other languages since most will require you to explicitly use the return
keyword.

nicolasverger3
3,451 PointsThanks a lot andren ! I agree. Using "return" adds clarity to the code. Having experience in C++ and Javascript, I find Ruby's forgiving nature really surprising. Thanks again andren, that was fast !
nicolasverger3
3,451 Pointsnicolasverger3
3,451 PointsThank you William, I found your comment really clear and helpful. I'll definitely read the Ruby Style Guide.
I probably got started with the wrong assumptions about code readability in Ruby even though I still think implicit return does not contribute to code clarity (particularly for beginners). But since my experience with Ruby is almost null (for now), I'll probably have time to change my view about that and learn to embrace Ruby's conventions :)