Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial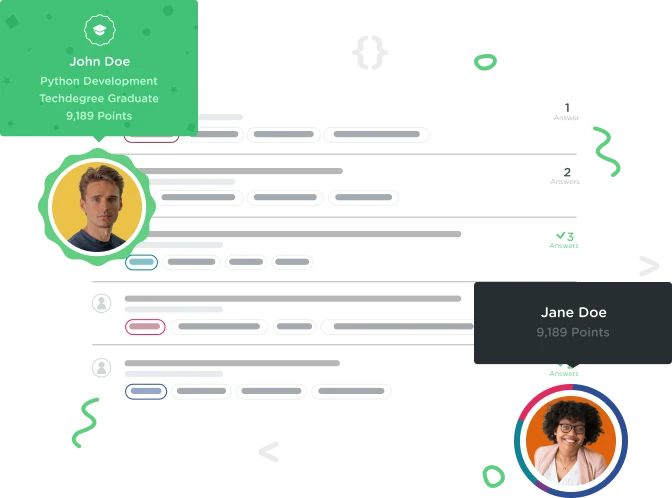

Teodor Jonsson
3,356 PointsWhere to start? Been sitting here for some time now not even knowing where to start with this..create new function?
I don't even know where to start, can't seem to find my way around this problem....
const languages = ['Python', 'JavaScript', 'PHP', 'Ruby', 'Java', 'C'];
const section = document.getElementsByTagName('section')[0];
// Accepts a language name and returns a wikipedia link
function linkify(language) {
const a = document.createElement('a');
const url = 'https://wikipedia.org/wiki/' + language + '_(programming_language)';
a.href = url;
return a;
}
// Creates and returns a div
function createDiv(language) {
const div = document.createElement('div');
const h2 = document.createElement('h2');
const p = document.createElement('p');
const link = linkify(language);
h2.textContent = language;
p.textContent = 'Information about ' + language;
link.appendChild(p);
div.appendChild(h2);
// Your code below
function
// end
return div;
}
for (let i = 0; i < languages.length; i += 1) {
let div = createDiv(languages[i]);
section.appendChild(div);
}
<!DOCTYPE html>
<html>
<head>
<title>Programming Languages</title>
<style>
body {
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>Programming Languages</h1>
<section></section>
<script src="app.js"></script>
</body>
</html>
3 Answers

Pat Goodwin
7,120 PointsNever mind. I got it. You have to see that the function linkify(language) was passed into the second function. That give you the link as 'a', but you cannot append 'a', since it does not exist as a const. The const is link written: const link = linkify(language);
Thus you must append as follows. div.appendChild(link);
This one stumped me for a while...
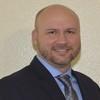
John Collins
Full Stack JavaScript Techdegree Graduate 28,593 Pointsconst languages = ['Python', 'JavaScript', 'PHP', 'Ruby', 'Java', 'C'];
const section = document.getElementsByTagName('section')[0];
// Accepts a language name and returns a wikipedia link
function linkify(language) {
const a = document.createElement('a');
const url = 'https://wikipedia.org/wiki/' + language + '_(programming_language)';
a.href = url;
return a;
}
// Creates and returns a div
function createDiv(language) {
const div = document.createElement('div');
const h2 = document.createElement('h2');
const p = document.createElement('p');
const link = linkify(language);
h2.textContent = language;
p.textContent = 'Information about ' + language;
link.appendChild(p);
div.appendChild(h2);
// Your code below
div.appendChild(link);
// end
return div;
}
for (let i = 0; i < languages.length; i += 1) {
let div = createDiv(languages[i]);
section.appendChild(div);
}

Cheo R
37,150 PointsWhenever I find myself in these types of situations, i try to break down what they're asking into smaller chunks:
This code should create a sequence of div elements that
each hold a headline above
and a link below.
Currently only the headlines display
and the links do not.
Can you figure out how to fix this to
make the links display under each headline?
If you look at the preview, you can see it displays the headlines. So that can be marked off. Looking at the code, it looks like the div element's also created. That can be marked off (sequence of div elements). Looks like all you need to do is add the missing link below.
You can also go through each bit of the code and explain to yourself what you think is happening, for example:
// languages to iterate over
const languages = ['Python', 'JavaScript', 'PHP', 'Ruby', 'Java', 'C'];
// grabs the section element found in index.html
const section = document.getElementsByTagName('section')[0];
// Accepts a language name and returns a wikipedia link
function linkify(language) {
// create an anchor tag
const a = document.createElement('a');
// make a url for that anchor tag
const url = 'https://wikipedia.org/wiki/' + language + '_(programming_language)';
// set the link target
a.href = url;
// return the anchor tag
return a;
}
// Creates and returns a div
function createDiv(language) {
// create div tag
const div = document.createElement('div');
// create h2 tag
const h2 = document.createElement('h2');
// create p tag
const p = document.createElement('p');
// get the anchor link for a given language
const link = linkify(language);
// set the language in the header
h2.textContent = language;
// add language info in the content
p.textContent = 'Information about ' + language;
link.appendChild(p);
div.appendChild(h2);
// Your code below
// what you want to do here is to append the link tag (that we made above)
// end
return div;
}
// for each language at a particular index in the language array
for (let i = 0; i < languages.length; i += 1) {
// create a new div tag for that language
let div = createDiv(languages[i]);
// add that div to section
section.appendChild(div);
}
Then you can figure out what needs to be done to get what they're looking for (wrote it in the comment above).

Pat Goodwin
7,120 PointsI am just not getting this quiz. I have tried several times, and I keep getting can't find variable a