Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial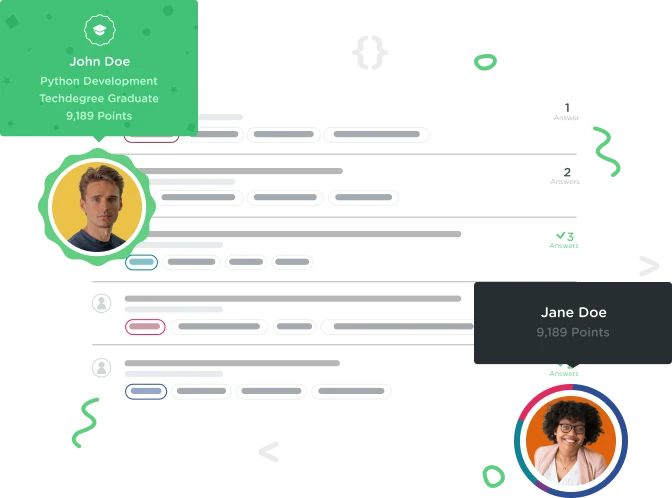

Dominique Robinson
6,504 PointsWhich condition is wrong and why?
I do not see why these conditions do not make sense to the conditions of the question.
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today == 'Friday' || money <= 9 ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

furkan
11,733 PointsSo, the question asks: Something's wrong with this script. The value in the variable money is only 9. But if you preview this script you'll see the "Time to go to the theater" message. Fix this script so that it correctly tests the money and today variables and prints out the proper alert message: "It's Friday, but I don't have enough money to go out"
To begin with:
1) The conditions they are testing contains a logical OR operator which looks like this || 2) The logical OR tests both variables, the money and today, and will run if one of those variables is true 3) This means, regardless of whether one of the variables is false and other is true, it will always run the code in those statements, so BOTH must be false in order to not run 4) This is not a good idea, so we must first change the use of OR operator to AND operator which looks like this &&
This is the code they have given you:
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Replace all the || with && in the first three conditions:
finally, look at the condition below that we want to run:
```javascript
else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
}
In this part, the condition is missing the money variable and contains a strictly NOT operator which looks like this: !===
We must add a money variable that tests for less than 10 && today must be 'Friday'. You can fix that like this:
else if ( money < 10 && today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
}
To conclude, your correct and final code should look like this:
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( money < 10 && today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
I hope that helped. Happy coding :)

Dominique Robinson
6,504 PointsHelped a ton. Thank you!

Teresa Balistreri
6,714 PointsHello Dominique.
I see 2 problems. 1) money = 10 is never dealt with. (actually, money greater than 9 up through 10 is not dealt with) 2) the || (or) statement could give a false alert.
} else if ( money > 10 && today === 'Friday' ) { alert("Time for a movie");
// what if the money is exactly 10? It will proceed to the next statement which also doesn't address 10.
} else if ( today == 'Friday' || money <= 9 ) {
/* I think this "else if" should use && (and) not || (or). Using || (or) it could either be that 1) it is Friday and you have <=9, (actually <=10), or 2) it is not Friday and you have <=9. */
alert("It's Friday, but I don't have enough money to go out");
// so it could be another day and not enough to go out,
// or Friday and 10 (should 10 be enough to go to the movie?)
So, it seems to me that there are 2 problems (or at least 1 problem):
If it isn't Friday and the money is <=9, the alert will be, "It's Friday, but I don't have enough money to go out", which will be the incorrect. It isn't Friday.
If it is Friday and money is 10, the alert will be "It's Friday, but I don't have enough money to go out". Did they intend that 10 is enough for a movie? In any case, 10 is never dealt with.