Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial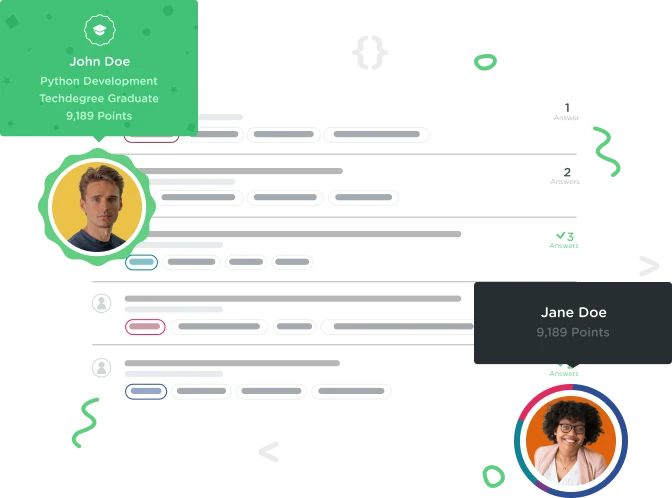

Matthew Kreiling
2,132 PointsWhich is more performant? .hide in jquery or display: none in css.
And does it make a huge difference? Assuming you are already using jquery.
3 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsI've been thinking about this question some more, and I figured I'd share some additional thoughts.
The jsPerf link that James Barnett provided is a good one, but it still relies on JavaScript. The element's display
properties are being changed through JavaScript code, so there's still a minuscule overhead there.
It's very hard to compare the speed of CSS (which relies on the rendering engine) and JavaScript (which relies on the JavaScript engine). A slightly better scenario would be comparing the page rendering speed of two identical sites (but where one hides an element with CSS and the other with JavaScript).
Still, since jQuery (or any other JS sane method of hiding elements) relies on display: none;
, the point is moot.
If you are truly worried about the performance, there's something else to consider. It's not how you apply properties to target elements, but rather how you target those elements.
CSS selectors (something that many JS libraries depend upon, including jQuery) have varying speeds. ID selectors are always the fastest. Universal selectors are slowest. Class and tag selectors are in the middle, although class selectors are a bit faster than tag selectors.
But even those speed differences are so minor that they can often be ignored.
There are some things you could do, that I consider to be good practice:
If you have a single element on the page that you do a lot of things with, give it an ID. Don't rely on classes only. If you're using jQuery (or something similar), cache the selector for that element:
var $myTargetDiv = $("#myTargetDiv");
// and then use $myTargetDiv instead of writing the selector every time you're doing things with that element
If you can (if the logic of your app/site allows it), chain the jQuery methods on the selector as much as possible.
$myTargetDiv.css('background', 'red').fadeOut('slow');
// instead of
$myTargetDiv.css('background', 'red');
$myTargetDiv.fadeOut('slow');
If elements have certain CSS properties on the page load, set those in CSS instead of JavaScript. If elements change their appearance due to user interactions, define those changes in a separate CSS class and then toggle that class on the element with jQuery, instead of applying properties (and removing them) with .css()
.
Both classes and IDs have their purpose, so don't be afraid of using them both.
Pseudo-class selectors can be very useful sometimes, but if you can do things without them, then don't use them. Very clever and complex selectors can often be hard to read (and usually will be slower).
And remember, even if you choose a "slower" version of doing something, the computers (and mobile devices) are so fast today that no one is likely to notice.

Dino Paškvan
Courses Plus Student 44,108 PointsjQuery's hide method actually sets the display
property of the element(s) it was called on to none
(among other things, like caching the old display
property of the element so it can be reset with .show()
). If you need elements hidden on the page when it loads (so you can display them later on due to some user action), it's always better to hide them with CSS.

James Barnett
39,199 Points4th result on google for "hide vs display none"
http://jsperf.com/jquery-show-hide-vs-css-display-none-block/27
James Barnett
39,199 PointsJames Barnett
39,199 PointsNot sure where you are going with this. Anyone who suggests not using IDs with JavaScript is talking crazy talk.
Dino Paškvan
Courses Plus Student 44,108 PointsDino Paškvan
Courses Plus Student 44,108 PointsIn the past, ID selectors were often misused and suddenly a lot of blogs started suggesting that IDs were evil and classes were the way to go, which is complete nonsense. Sadly, those articles can still be found on the web and plenty of people follow those suggestions without checking the facts.
Matthew Kreiling
2,132 PointsMatthew Kreiling
2,132 PointsAs a CSS person, I rarely use IDs for styling because they are too specific - they cause very verbose selectors down the road if the styles need to be overridden. I know this topic has gone a little for from the original question.
It is interesting to learn that IDs are preferred selectors in JS.
Thank you both.