Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial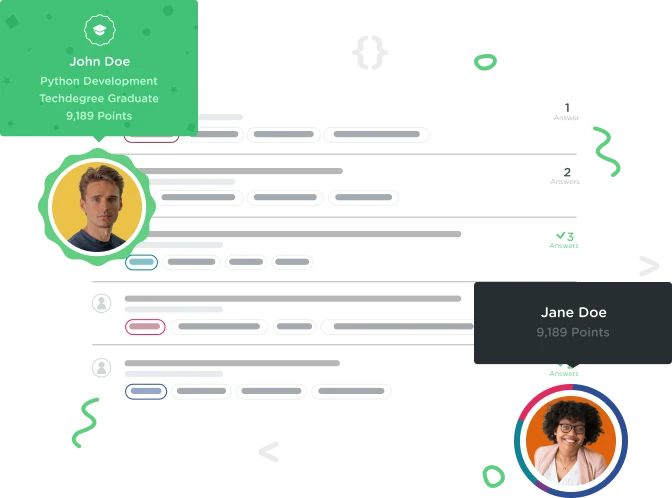

Carrington Seawright
Full Stack JavaScript Techdegree Student 8,226 PointsWhich line of code assigns li elements the className 'responded' for the' if' condition statement?
The code is working fine but I'm trying to figure out where exactly the line of code is that assigns li elements the 'responded' className so that they are able to be identified in the 'if' statement, nested in the 'for' loop of the 'if(isChecked)' condition.
const form = document.getElementById('registrar');
const input = form.querySelector('input');
const mainDiv = document.querySelector('.main');
const ul = document.getElementById('invitedList');
const div = document.createElement('div');
const filterLabel = document.createElement('label');
const filterCheckBox = document.createElement('input');
filterLabel.textContent = "Hide those who haven't responded";
filterCheckBox.type = 'checkbox';
div.appendChild(filterLabel);
div.appendChild(filterCheckBox);
mainDiv.insertBefore(div, ul);
filterCheckBox.addEventListener('change', (e) =>{
const isChecked = e.target.checked;
const lis = ul.children;
if(isChecked){
for (let i = 0; i<lis.length; i++){
let li = lis[i];
if (li.className === 'responded'){
li.style.display = '';
} else {
li.style.display = 'none';
}
}
} else {
for (let i = 0; i<lis.length; i++){
let li = lis[i];
li.style.display = '';
}
}
});
function createLI (text){
const li = document.createElement('li');
const span = document.createElement('span');
span.textContent = text;
li.appendChild(span);
const label = document.createElement('label');
label.textContent = 'Confirmed';
const checkbox = document.createElement('input');
checkbox.type = 'checkbox';
label.appendChild(checkbox);
li.appendChild(label);
const editButton = document.createElement('button');
editButton.textContent = 'edit';
li.appendChild(editButton);
const removeButton = document.createElement('button');
removeButton.textContent = 'remove';
li.appendChild(removeButton);
return li;
}
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = "";
const li = createLI(text);
ul.appendChild(li);
});
ul.addEventListener('change', (e) => {
const checkbox = event.target;
const checked = checkbox.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked){
listItem.className = 'responded';
}else{
listItem.className = '';
}
});
ul.addEventListener('click', (e) => {
if (e.target.tagName == 'BUTTON'){
const button = e.target;
const li = button.parentNode;
const ul = li.parentNode;
if (button.textContent === 'remove'){
ul.removeChild(li);
} else if (button.textContent === 'edit'){
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'save';
} else if (button.textContent === 'save'){
const input = li.firstElementChild;
const newName = document.createElement('span');
newName.textContent = input.value;
li.insertBefore(newName, input);
li.removeChild(input);
button.textContent = 'edit';
}
}
});
There's code that does this within the ul event listener but if i'm correct it's only accessible within that function's scope.
Any help is greatly appreciated.
Best,
1 Answer
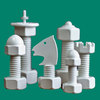
Steven Parker
230,274 PointsIt's the class name of a DOM element that's being updated, and the entire DOM is global. The local variable, which as you noticed is limited in scope, only serves as a reference to the element while the attribute is being updated.
prasadrane
571 Pointsprasadrane
571 PointsIn this function the variable being updated is not local variable; it is reference to actual listItem from
event.target.parentNode.parentNode
Thus value className gets updated for the listItem here.