Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial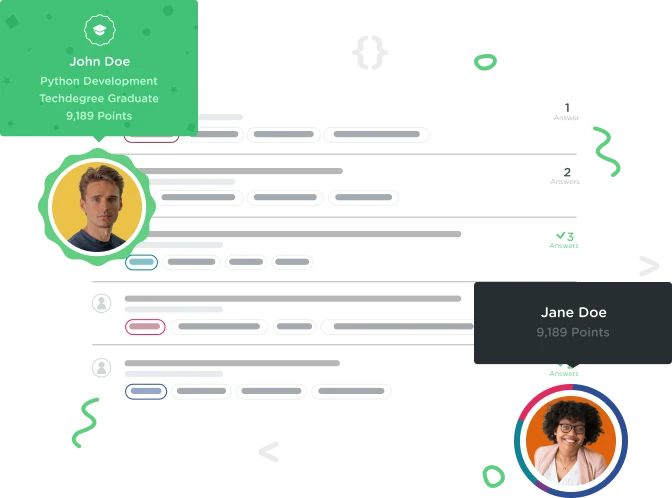

Anon nas
10,193 PointsWhile being stuck, I found another solution. Please evaluate my code. Thanks!
function getRandomNumber( lower, upper ) { if (isNaN(lower) || isNaN(upper) ) { alert("Sorry, your input may only contain numbers, and can not be left blank. Please try again."); process.exit(); } else { return Math.floor(Math.random() * (upper - lower + 1)) + lower; } }
alert( getRandomNumber( 9, 24 ) ); alert( getRandomNumber( 1, 100 ) ); alert( getRandomNumber( 200, 'five hundred' ) ); alert( getRandomNumber( 1000, 20000 ) ); alert( getRandomNumber( 50, 100 ) );
2 Answers

Antti Lylander
9,686 PointsIt would make more sense to me like this:
function getRandomNumber( lower, upper ) {
if (isNaN(lower) || isNaN(upper) ) {
alert("Sorry, your input may only contain numbers, and can not be left blank. Please try again.");
} else {
alert(Math.floor(Math.random() * (upper - lower + 1)) + lower);
}
}
getRandomNumber( 9, 24 );
getRandomNumber( 1, 100 );
getRandomNumber( 200, 'five hundred');
getRandomNumber( 1000, 20000 );
getRandomNumber( 50, 100 );

Antti Lylander
9,686 PointsI removed the process.exit() that is not defined (in browser at least) nor needed.
You had some inconsistency with alert and return. "Sorry.." was alerted and random number was returned. I chose to use alert for both as you can then just call the function at the end of your code.

Antti Lylander
9,686 Pointsnow, can you make it ask the lower and upper limits and then show the random numbers? I'll show you my solution then.

Raul Cisneros
7,319 Pointsfunction randomNumber(a, b) {
if (isNaN(a) || isNaN(b)) {
throw new Error("One or both numbers in your function call is not a number"); // console error msg
}else{
var num = Math.floor(Math.random() * (a - b -1) + (b + 1));
}
document.write(num); //code test
return num;
}
console.log(randomNumber(23, "one")); //function call and code test
This is what I came up with. Hope this helps. If I messed up please let me know so I can learn from my mistakes.
Adam Beer
11,314 PointsAdam Beer
11,314 PointsCode
Wrap your code with 3 backticks (```) on the line before and after. If you specify the language after the first set of backticks, that'll help us with syntax highlighting.