Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial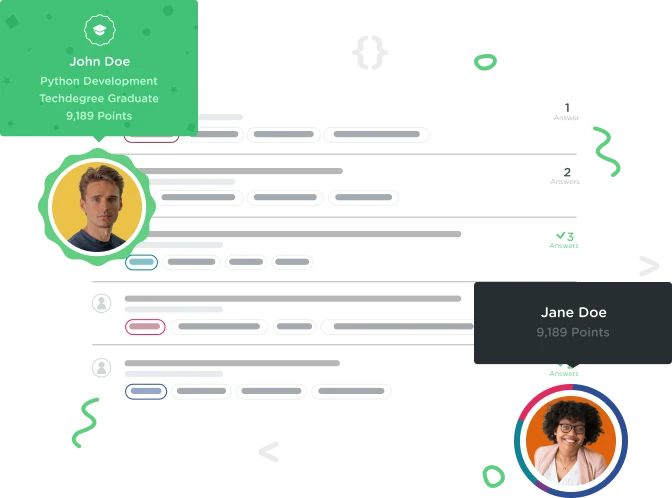

JAH Resink
360 Pointswhile loop
var count = 0 while ( count < 26 ) { document.write(count); count +1; }
what is wrong?
var count = 0
while ( count < 26 ) {
document.write(count);
count +1;
}
2 Answers
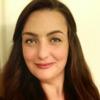
Jennifer Nordell
Treehouse TeacherHi there! You've inadvertently created an infinite loop. Although you're adding 1 to count each time, you're never actually assigning the value back into count. Here's three alternatives:
count += 1;
count++;
count = count + 1;
All three of these lines do the same thing. They take the count, add one to count, and then place the resulting value back in as the new value of count.
Hope this helps!

Luke Pettway
16,593 PointsYou are creating an infinite loop. count + 1 isn't incrementing the count but taking 0 and adding 1 to it. So each iteration is 1.
var count = 0
while ( count < 26 ) {
document.write(count);
count +1;
console.log(count +1);
}
Will output like this: 1 1 1 1 1 1 1
When you are incrementing a number by one you can opt to do this instead:
var count = 0
while ( count < 26 ) {
document.write(count);
count++; //<-- This equates to count = count+1
}