Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial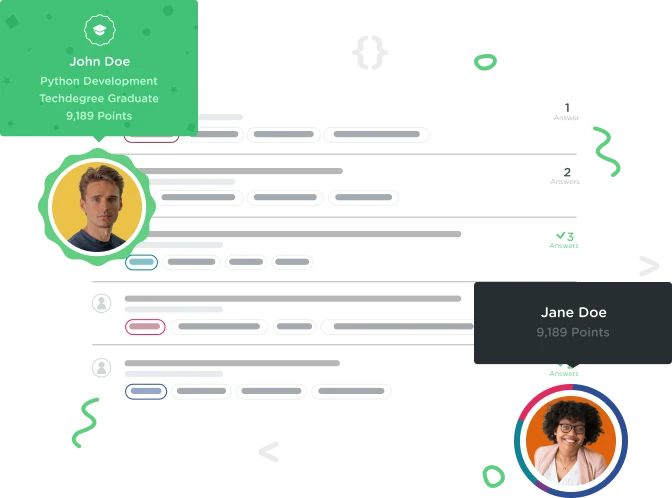

Brian Uribe
3,488 PointsWhile Loop
I kind of just memorized how to do this, but I need help with understanding how this statement works.
when I have print(sum += numbers[counter])
how does the loop know to add the counter to the numbers? What is going on in this loop exactly? I know that i'm saying to print sum (which is zero) but then add the numbers to the sum, six times. But where does counter come into play? Why do I need it there? What is its role? Shouldn't I just need the counter++ to stop the loop from going on forever?
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
3 Answers
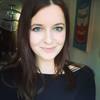
Marina Alenskaja
9,320 PointsHi
I'll do my best to try to explain this. So you have your "numbers" array. To iterate over them, we want to move 1 index point each time, since arrays are ordered lists with index numbers. The "counter" variable starts at 0 - which is also the first index number in arrays. Each time, the loop runs the code, retrieves the number in the array at the index of the counter, and then adds 1 to counter. That means next time the loop runs, the index number is 1, and the number in the array at index 1 is retrieved. When the "counter" variable hits 7, the array won't have any item, and the loop will therefore stop running.
I hope that makes sense. Happy coding :-)
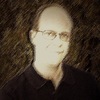
Jason Anders
Treehouse Moderator 145,860 PointsHey Brian,
In that statement, the variable counter
will be replaced with the current iteration of the loop. The value being placed there is the index number that is used to access the numbers array
. Throughout the loop, you will get
print(sum += numbers[0]) //first number in the array
print(sum += numbers[1]) //second number of the array ... and so on
print(sum += numbers[2])
print(sum += numbers[3])
print(sum += numbers[4])
print(sum += numbers[5])
print(sum += numbers[6])
With each iteration, the next number in the array is accessed with the index and then added to the variable
.
So counter++
is all that is needed to prevent the loop from running forever, but it is also being used as the index number to access the entire array.
Hope that makes sense. Keep Coding! :)

Brian Uribe
3,488 PointsSo this is my code
let numbers = [2,8,1,16,4,3,9] var sum = 0 var counter = 0
while counter < numbers.count { print(sum += numbers[counter]) counter++ }
with sum, am I actually adding all the numbers together? Shouldn't playground print sum as whatever all the numbers add up to? Because playground is just giving me (7 times) meaning it ran the loop 7 times. I guess what i'm really asking is what is the purpose of this code other than to answer the challenge question?
another question on the counter variable, shouldn't the line while counter < numbers.count and counter++ be enough to let the code know that it should stop after it has run 7 times? Why do I need to embed counter in the body of the code like this: {print(sum += numbers[COUNTER])?
Thanks for the help guys.
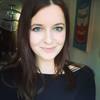
Marina Alenskaja
9,320 PointsThe purpose of the code is to add the numbers together and store it in the sum variable. In xcode, you can click on the little plus sign to see the result - it just says 7 times as default, to show you that the loop ran 7 times.
Seems like you didn't finish the second question? :-)

Brian Uribe
3,488 PointsI finished, I just wanted to make sure I fully understood what I was doing though. Thank you!
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsHey Marina,
You type a bit faster than I. Lol.
I think that's a pretty great and clear explanation.
Marina Alenskaja
9,320 PointsMarina Alenskaja
9,320 PointsHi Jason
Haha, thank you and you too!