Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial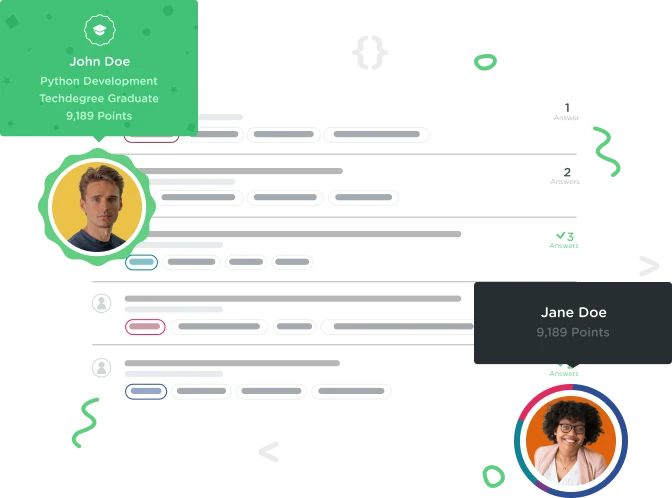

Eric Shelby
28,300 PointsWhile loop in python
I am having trouble with his challenge. The instructions state: "Make a while loop that runs until start is falsey.
Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99.
If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number.
Finally, decrement start by 1."
The problem is that the challenge keeps telling me that I'm getting the wrong number of prints. I'm not use to while loops in Python. So, I don't fully understand their mechanics. an explanation would be nice.
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while False:
number = random.randint(1,99)
if even_odd(number) == 0:
print("{} is even".format(number))
else:
print("{} is odd".format(number))
start = start - 1
5 Answers
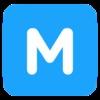
merc1er
6,329 PointsYou wrote while False
, which is not coherent since what is inside this while will not be taken into account.
Try:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start: # which is the same as while start != False
number = random.randint(1,99)
if even_odd(number) == 0:
print("{} is even".format(number))
else:
print("{} is odd".format(number))
start = start - 1

Eric Shelby
28,300 PointsThanks for all who responded. I now realize that because the while loop has a countdown, a tradition true-false trigger wouldn't work in this case. So, adding start instead fixed things. I worked with while loops with other programming languages. The way Python does while loops is new to me. Hopefully, I get the hang of things.

Sarah Colby
Courses Plus Student 1,224 PointsWhat was your working code? My code below is saying Task 1 no longer works:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
rand_num = random.randint(1, 99)
if even_odd(rand_num):
print("{} is even").format(rand_num)
else:
print("{} is odd").format(rand_num)
start -= 1```

Eric Shelby
28,300 PointsSarah, I looked through your code and discovered it was a simple mistake: you simply added the ending parentheses to your "print" statements to the wrong areas. They ended to come after the format ending parentheses. They should be: print("{} is even".format(rand_num)) print("{} is odd".format(rand_num)) Easy mistake to miss. It happens to me a lot.
I should also point out that you have forgotten to add "== 0" to your "if" statement, making it: if even_odd(rand_num) == 0: It what allows the "if" statement to filter through the even and odd numbers generated.
Furthermore, the "even_odd" method inverts the results of "num % 2". So, instead of even numbers producing a result of zero, odd numbers would. So, you need to switch the print statements in the "if" and "else" blocks to get a right answer. Everything else appears to be fine.

Eric Shelby
28,300 PointsSarah, I goofed a little. Apparently, your statement "start -=" isn't correct. I don't know that exact way it should be written. I would just write "start = start - 1" to just save yourself the headache.

Eric Shelby
28,300 PointsSarah, ignore the last post. "start -= 1" is correct. I was the one who goofed. Sorry about that.
Luc de Brouwer
Full Stack JavaScript Techdegree Student 17,939 PointsLuc de Brouwer
Full Stack JavaScript Techdegree Student 17,939 Pointswhile loops usually require you to tell the loop to run (true) until criteria is met, when criteria is met return false, so you break out of the loop.