Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial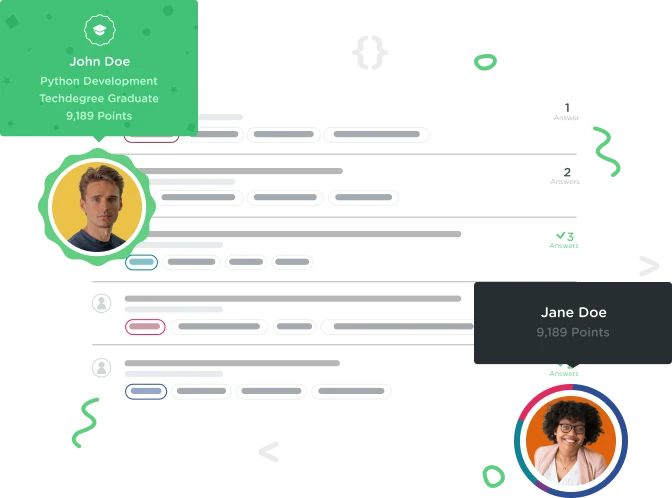

Justine Lam
8,785 PointsWhile loop vs For loop
Why would I used a while loop instead of a for loop in real life?
2 Answers

Stone Preston
42,016 Pointssometimes one loop does the job better than the other. For instance if you were making a game and needed to make the game loop over and over, you could use a while loop that would run infinitely by using
while (i = 1) {
and then never changing i. or if you only wanted to do something while the user was using the mouse you could use something along the lines of
while (mouseIsMoving()) {
For loops (and for each loops especially) are definitely more common, but sometimes you just need a while loop to get the job done.
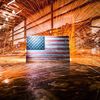
Andrew Shook
31,709 PointsYou would use a while loop to loop through code while some condition is true (like boolean true). For instance, my old game where built using a do...while loop. So the code would run once (start the level), and while (playerAlive == true) the main game loop would keep going ( this is how something like the original Super Mario was programmed).
A for loop is mostly used to loop through a piece of a given number of time ( the length of an array or x number of time /5). Note that the condition for a for loop must still be true, but the condition is usually testing a numeric value and not a boolean. Although while loops can also test numerics.

Justine Lam
8,785 PointsNeat, I didn't know that about while loops being able to handle booleans. Why can't for loops handle them? Is it because of how for loops are constructed?
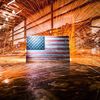
Andrew Shook
31,709 PointsYes and no. Technically, for loops can handle a boolean. In fact, you can write a for loop like this:
for(;;;){
///do something
}
This will cause an infinite loop. You can also write a for loop like this:
for( ; true; ;){
//do something
}
This will also cause an infinite loop since there is nothing to that wil ever set the condition to false. Finally, building off that last example you could write a for loop like this:
bool test = true;
int i = 0
for ( ; test; ){
//do something
if( i == 5){
test = false;
}
i++;
}
This code will work ( this is just sudo-code so it would not actually work), but you had to declare two variable to make the loop when you could have just done this:
for( i = 0; i =< 5; i++){
// do something
}
As you can see, you can use a boolean in a for loop but its not really practical. Its more practical to loop a boolean in a while loop:
bool playerAlive = true;
function checkCollission(){
if ( didCollideWithNPC()){
playerAlive = false;
}
}
do{
// main code for super mario
// calls all the functions to control movement, graphics and sound effects
checkCollision();
}while(playerAlive); //this part of the loop will keep the game going until the player hits an NPC then the game stops.
Andrew Shook
31,709 PointsAndrew Shook
31,709 PointsOut posted me by seconds. Darn you meddling MODS!
bronnyreinhardt
1,007 Pointsbronnyreinhardt
1,007 PointsHi, I had the same question as Justine but I'm still curious about the difference between a for and a while loop.
I understand that the for loop is for 'making counter-controlled loops', as the preamble to the lesson states.
In the preamble to the while loop lesson, the preamble states that it's for 'executing a block of code without providing any construct for keeping a counter'.
I think my confusion stems from the fact that both code examples have the same type of conditional expression ('If the indexing variable is less than three ...') so I can't see how they're different.
What part of the for example used in the for loops lesson, exactly, is the 'construct [that keeps] a counter'?
Andrew Shook
31,709 PointsAndrew Shook
31,709 PointsMilly Main, the variable inside the parentheses of the for loop, usually "i" in most examples, is the construct that keeps the counter. So in this for loop:
$i = 0 initializes the counter, $i less than 20 check the condition of the counter, and $i ++ increments the counter when appropriate.
bronnyreinhardt
1,007 Pointsbronnyreinhardt
1,007 PointsThanks Andrew Shook