Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial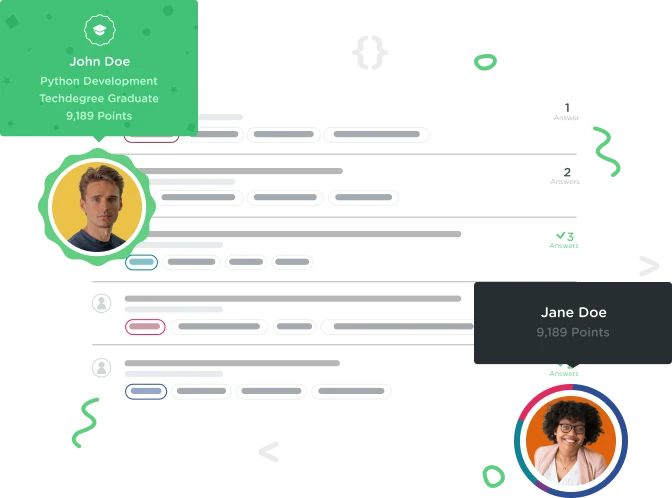

Michael Southon
1,951 PointsWhile Loops
I'm getting this error message when I give the following response to this challenge: You need to use 'println()'
let index = 0 while numbers.count < 9 { println(numbers[index]) }
I am using the 'println()' command so I'm puzzled by this error message.
Can anyone help?
Thanks in advance!
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
let index = 0
while numbers.count < 9 {
println(numbers[index])
} // You need to use 'println()'
3 Answers

Gunhoo Yoon
5,027 PointsI haven't had a chance to start Swift course but I think there is some logic error in your code. But I think I know where you made mistake.
So let me first correct your mistakes but from second.
//Second mistake
while numbers.count < 9 {
println(numbers[index])
}
1 Your loop won't actually run because numbers.count
has 10 elements. Probably that's why the compiler didn't recognize your println() since it never reached that state.
2 Even adjusting your loop condition to number.count < 11, 12 .... whatever
it will only result infinite loop because number.count
never changes. So it is either broken loop or indefinite loop.
3 Even if your loop did run, it will only printout numbers[0] because you've never increased the index in your code.
//First mistake.
let index = 0
4 From what I've searched on Stackoverflow let
is a constant which can't be changed. source. So even if you increased the index
you would get error because it cannot be changed. So you need to use var
like normal variable.
I went from second to first because that's what was actually causing You need to use 'println()'
This is the working version.
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
//Use variable that is change-able.
var index = 0
//The index will start from 0, will increase every iteration, so it will eventually end.
while index < numbers.count {
//print first because you start from index 0
println(numbers[index])
//this does this incrementing
index++
}

Unsubscribed User
3,801 PointsI have never done Swift before, but I will try to explain the problems I see with your code.
1) You are using the keyword let
for your index
, which means you are declaring index
as a constant variable. This will mean that further down in your code, you will not be able to modify index
.
2) Your while
loop will never iterate. The condition for your while
is saying 'loop while numbers.count
is less than 9'. However, numbers.count
has a total of 10 items, but the count will return '9' (because the array index starts at 0). This is the same as checking if 9 is less than 9, which will always be false.
3) Inside of your loop, you are only calling println
. In order for a while
loop to iterate through your array, you would need to add a variable which is either incremented or decremented on each loop. It looks like you were going to use index
for this, but it wasn't implemented correctly.
I came up with the following solution which passes the code challenge:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
var index = 0
while index < numbers.count {
println(numbers[index])
++index
}
This will loop through each item in the array and print the value. At the end of each loop, ++index
is adding 1 to the index
variable, which is helping us with our iteration through the array. For this to work correctly, I had to declare index
with var
and not with let
.
Hope this helps!

Michael Southon
1,951 PointsThanks to you both! Your responses really helped me understand what was going on. Thanks for your patience with a beginner :-)