Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial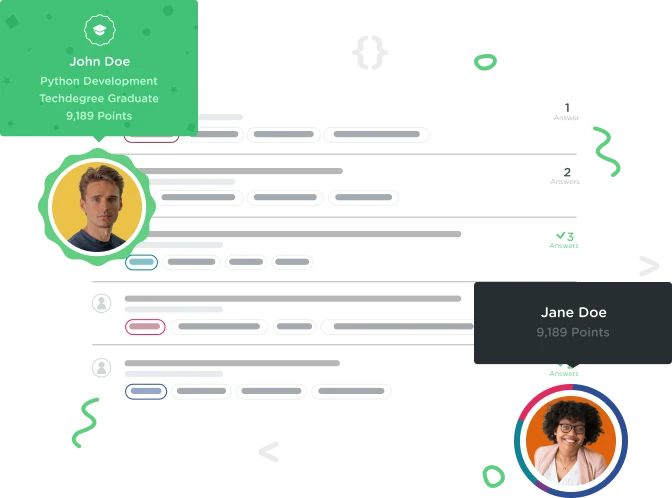

ROB TILLEY
842 PointsWhile Loops question.
var todo: [String] = ["Return Calls","Write a blog post","Cook dinner","Pickup Laundry","Buy bulbs"]
var index = 0
while index < todo.count
{
println(todo[index])
index++
}
I understand how most of the loop works, however I can't figure out how the println() function works. How does it make the connection to printing the physical list by looking at the variable "index". I see that while the index is still less than the total number of items in the array, "todo", it will initialise the print function. But don't understand how (todo[index]) makes works. Difficult to explain in text. Sorry.
1 Answer
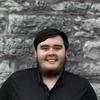
Michael Hulet
47,912 PointsWhat the line "todo[index]
" does is get the object in the array at the position of the index
variable. Let's walk through the loop step by step. To reiterate, here's the code you posted, plus some comments to explain line-by-line:
//Makes an Array of Strings
var todo: [String] = ["Return Calls", "Write a blog post", "Cook dinner", "Pickup Laundry", "Buy bulbs"]
//Creates an Int variable and sets it to 0
var index = 0
//Loops the code in the brackets while the value of the index variable is less than the number of items in the todo Array
//This ensures that the code in the block will never run when the index variable is set to a number that isn't a valid index of the todo Array
while index < todo.count{
//Grabs the String in the todo array at the position in the array of the current value of the index variable and prints it out
println(todo[index])
//Adds one to the index variable
index++
}
The first time this code runs, the todo
variable will be created, as well as the index
variable. The loop will run for the first time, with index
set to 0
. When it gets to the call to println
, it will grab the String
at position 0
in the todo
array. Position 0
represents the first object in the array. In this case, the first object is "Return Calls"
, so "Return Calls" will be printed to the console. Once that is done, index
is incremented, and the loop runs again.
The second time the loop runs, index
is set to 1
. When it gets to the println
statement, it will grab the String
at position 1
in the todo
array, and print it to the console. Position 1
represents the 2nd object in the array, so "Write a blog post" will be printed.
I guess the takeaway you're supposed to get with this is that the way you get objects out of an Array
is by asking for it like this:
let object = array[position - 1]
The code you made will quickly grab every object from the array in order and print it to the console. Because the condition of the loop is to run while the index
variable is less than the total number of objects in the todo
array, index
will never hold a number that doesn't represent an object in the todo
array, and the loop will stop when it does.
ROB TILLEY
842 PointsROB TILLEY
842 PointsThanks Michael. You explained it very well. I will use the explanation for my notes. I assume then that println(todo[index]) links the two variables together somehow so that when the print function is activated it looks for the item in the todo array that matches the current value of the variable index, then prints it?????
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsCorrect. When you nest function calls like that, they work from the inside out. That way, what it does first is grabs the string that resides at the position in the
todo
array of the current value of theindex
variable, then it hands that string to theprintln
function, whose job is to print it to the console. If you wanted to expand it a little to make it more clear, you could rewrite the loop like this:ROB TILLEY
842 PointsROB TILLEY
842 PointsThanks again Michael. You're a champion. It makes sense now. Rob
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsIf you wanna be super-verbose, the code you wrote would be equivalent to doing this:
That code works, but there's a few problems with it. Mainly that problem is that you have to write
println(todo[])
over and over and over again. Another problem is that if you ever add or remove anything from thetodo
array, you have to add or remove lines ofprintln
statements. It's a lot easier, more maintainable, and safer to work with to just make a loop that prints out everything in the arrayMichael Hulet
47,912 PointsMichael Hulet
47,912 PointsNo problem! I'm glad I could help