Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial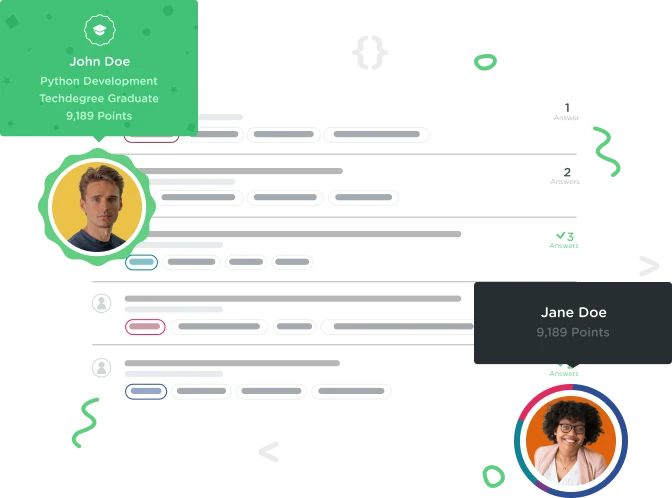

Alexander Oaktree
515 PointsWhile taking this challenge should I still insert an if statement? Knock Knock
I believe I should but not really sure!
3 Answers
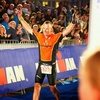
Steve Hunter
57,712 PointsHi Alexander,
An if
statement could certainly check that a test is passed, yes. However, it is not a "loop" - the test happens only once.
The question says:
We want to move that prompting code into a do while loop. Wrap the code into a do while code block and check in the while condition to see if who is "banana" so that the loop continues.
So, we need a do ... while
loop, it says. We have three lines of code already to help us:
// Person A asks:
console.printf("Knock Knock.\n");
// Person B asks and Person A's response is stored in the String who:
String who = console.readLine("Who's there? ");
// Person B responds:
console.printf("%s who?\n", who);
So, we need to deal with the first scenario - the answer being "banana". This means we need to loop back through the whole knock-knock sequence. The sequence must run at least once before the test can be applied. This is why the do ... while
loop is best for this purpose.
do {
console.printf("Knock Knock.\n");
String who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while (who.equals("banana"));
This takes you through the required sequence of asking the question and getting the answer, it then loops back to the beginning if the answer given is equal to "banana". This will carry on as long as that answer is given.
That's not all, though ... the hint given let's us aware of this. There's a scoping issue:
HINT: Remember to move your who declaration outside of the do block so that it can be accessed.
All that means is that the initial declaration of the variable who
needs to happen before the inside of the loop. Like this:
String who;
do {
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while (who.equals("banana"));
That should work for you now.
Steve.
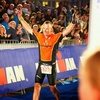
Steve Hunter
57,712 PointsHi Alexander,
Have a look through the following thread and see if that helps. If not, just shout and I'll see where you're at.
Steve.

Alexander Oaktree
515 PointsNot really that helpful. I am a noob in programming and just started taking the android developement track, so just getting started with Java.
So, the challenge requires to create a loop as long as the answer is Banana. So, shouldn't I insert an if statement, in order to create the loop as long as the answer is Banana?
Moreover, I understand that the answer we are expecting is Orange, but the course has not yet addressed this issue (that is, waiting for the user to give us a specific set answer to a given question), so creating a loop AS LONG AS the answer is not Orange would be asking us for something we haven't studied yet.
In a nutshell, I only need to understand the most basic scenario! lol ;)
Sorry to bother you mate!

MUZ140265 Blessing Kabasa
4,996 Pointsthe if statement can be used in the second task not the first one. for the first one you can do as follows. String who;
do {
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while (who.equalsIgnoreCase("banana"));
but for the second task you can use the if statement as follows
if (who.equalsIgnoreCase("orange")){
console.printf("%s i am glad you are not another Banana", who);
}
Alexander Oaktree
515 PointsAlexander Oaktree
515 PointsYep! That worked! Cheers
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsCool - the main thing is making sure you understood it, though. My explanations can be a bit pants at times.
If you have any questions, just shout!
Steve.