Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial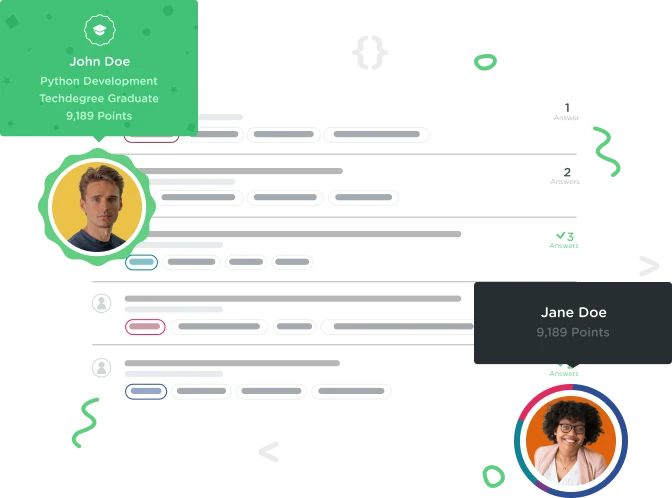

adrian garcia
4,658 PointswhileLoops.js
Here's my line of code that I had trouble on.
// 1. Write a while loop to build a string of numbers from 0 to 4,
// separated by spaces, and store the string in the variable text
.
print('1st Loop:');
text = '';
// Write 1st loop here:
let i = 0;
while( i <= 4) {
text += i + ' ';
i += 1;
}
print(text); // Should print 0 1 2 3 4
.
// 2. Write a while loop to build a string of numbers from 1 to 5,
// separated by spaces, and store the string in the variable text
.
print('2nd Loop:');
text = '';
// Write 2nd loop here:
let I = 1; while ( I <= 5) { text += I + ' '; I += 1; }
print(text); // Should print 1 2 3 4 5
.
// 3. Write a while loop to build a string of numbers from 5 to 1,
// separated by spaces, and store the string in the variable text
.
print('3rd Loop:');
text = '';
// Write 3rd loop here: let i = 0; while (i >= 1) { text += i + ' '; let i -= 1; }
print(text); // Should print 5 4 3 2 1
.
// 4. Write a while loop to build a string of numbers from 5 to 50--by 5's.
// The numbers should be separated by spaces, and stored in the variable text
.
print('4th Loop:');
text = '';
// Write 4th loop here:
print(text); // Should print 5 10 15 20 25 30 35 40 45 50
.
// 5. BONUS CHALLENGE: Write a while loop that builds a string of random integers // between 0 and 9. Stop building the string when the number 8 comes up. // Be sure that 8 does print as the last character. The resulting string // will be a random length. print('5th Loop:'); text = '';
// Write 5th loop here:
print(text); // Should print something like 4 7 2 9 8
, or 9 0 8
or 8
.
// Feel free to ignore this print function. It just formats the output a bit. function print(text) { console.log(text); if (!text.endsWith(':')) { console.log(''); }
I know the solution now, but I was very confused on why we couldn't use the let variable to define what ' i ' was. I only have come to a solution, because I looked at the solutions video, but I was just wondering if someone would care to explain to me what is going on if we define the ' i ' with the let variable.
1 Answer

Kristian Rosenqvist
6,037 PointsYou can only declare a variable once, unless it's part of a separate scope (like a for loop or a function). Once it has been declared to exist in a certain scope, you can reassign it or initialize it, but if you try to redeclare it in the same scope you get an error.
In your case you have declared i in the global scope before a while loop, and then you try to redeclare it later before a second while loop, which gives you an error.
For example, the following code would be acceptable:
let i;
i=0;
while (condition1) {}
i=0
while (condition2) {}
This is actually what happens under the hood when you do something like:
let i=0;
while (condition1) {}
i=0;
while (condition2) {}
Javascript automatically moves all variable declarations to the top of the scope like seen in the first code example.
Here's an example of a case where it would be acceptable to declare i twice.
let i=1;
function returnNumber() {
let i =2;
return i;
}
console.log(i + returnNumber());
//Output is: 12
The reason this works is becase i exists as a separate variable in the global scope and the function scope. If we had left out "let" in the function, we would have accessed and changed the global variable i instead.
I hope this was of some help. If you want to learn more about this you should read about Javascript variable scoping.